32. ChatGPT에게 물었습니다.
auth.onAuthStateChanged() 설명해줘.
The auth.onAuthStateChanged() method is a crucial part of Firebase Authentication. It is an event listener that triggers whenever there is a change in the authentication state of the user. This can include events such as a user signing in, signing out, or the current user's ID token being refreshed.
auth.onAuthStateChanged() 메소드는 Firebase 인증의 중요한 부분입니다. 사용자의 인증 상태가 변경될 때마다 트리거되는 이벤트 리스너입니다. 여기에는 사용자 로그인, 로그아웃 또는 현재 사용자의 ID 토큰 새로 고침과 같은 이벤트가 포함될 수 있습니다.
Key Points About auth.onAuthStateChanged()
auth.onAuthStateChanged()에 대한 핵심 사항
- Event Listener: It listens for changes in the authentication state. Whenever the authentication state changes, the provided callback function is executed.
이벤트 리스너: 인증 상태의 변화를 수신합니다. 인증 상태가 변경될 때마다 제공된 콜백 함수가 실행됩니다. - Callback Function: The callback function receives a user object if the user is signed in, or null if no user is signed in.
콜백 함수: 콜백 함수는 사용자가 로그인한 경우 user 객체를 수신하고, 로그인한 사용자가 없는 경우 null 객체를 수신합니다. - Real-time Updates: It provides real-time updates to your application about the user's authentication state.
실시간 업데이트: 사용자 인증 상태에 대한 실시간 업데이트를 애플리케이션에 제공합니다.
Usage 용법
You typically use auth.onAuthStateChanged() to maintain the authentication state of your application and to react to changes in the user's status. This is particularly useful for ensuring that user-specific data and UI elements are correctly displayed based on the user's login status.
일반적으로 auth.onAuthStateChanged()를 사용하여 애플리케이션의 인증 상태를 유지하고 사용자 상태 변경에 반응합니다. 이는 사용자 로그인 상태에 따라 사용자별 데이터 및 UI 요소가 올바르게 표시되도록 하는 데 특히 유용합니다.
Example
Let's go through an example to illustrate how auth.onAuthStateChanged() can be used within a Vue.js application using Vuex.
Vuex를 사용하여 Vue.js 애플리케이션 내에서 'auth.onAuthStateChanged()'를 어떻게 사용할 수 있는지 예시를 통해 살펴보겠습니다.
Step-by-Step Implementation
단계별 구현
- Firebase Initialization: Make sure Firebase is properly initialized in your project.
Firebase 초기화: 프로젝트에서 Firebase가 올바르게 초기화되었는지 확인하세요.
firebase.js
import firebase from 'firebase/compat/app';
import 'firebase/compat/auth';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
firebase.initializeApp(firebaseConfig);
const auth = firebase.auth();
const googleProvider = new firebase.auth.GoogleAuthProvider();
export { auth, googleProvider };
1. Vuex Store Module: Update your Vuex store to handle authentication state changes.
Vuex 스토어 모듈: 인증 상태 변경을 처리하도록 Vuex 스토어를 업데이트합니다.
store/modules/auth.js
import { auth, googleProvider } from '../../firebase';
const state = {
user: null,
error: null
};
const mutations = {
setUser(state, user) {
state.user = user;
},
setError(state, error) {
state.error = error;
}
};
const actions = {
async googleLogin({ commit }) {
try {
const result = await auth.signInWithPopup(googleProvider);
const user = result.user;
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async logout({ commit }) {
await auth.signOut();
commit('setUser', null);
commit('setError', null);
},
fetchUser({ commit }) {
auth.onAuthStateChanged(user => {
if (user) {
commit('setUser', user);
} else {
commit('setUser', null);
}
});
}
};
const getters = {
user: state => state.user,
error: state => state.error
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
2. Main App Component: Use the fetchUser action to initialize the authentication state when the app is created.
기본 앱 구성 요소: 'fetchUser' 작업을 사용하여 앱이 생성될 때 인증 상태를 초기화합니다.
App.vue
<template>
<v-app>
<v-main>
<v-container>
<v-row>
<v-col cols="12">
<google-login />
<v-btn color="primary" @click="logout" v-if="user">Logout</v-btn>
<p v-if="user">Logged in as: {{ user.email }}</p>
</v-col>
</v-row>
</v-container>
</v-main>
</v-app>
</template>
<script>
import { mapActions, mapGetters } from 'vuex';
import GoogleLogin from './components/GoogleLogin.vue';
export default {
name: 'App',
components: {
GoogleLogin
},
computed: {
...mapGetters('auth', ['user'])
},
methods: {
...mapActions('auth', ['fetchUser', 'logout'])
},
created() {
this.fetchUser();
}
};
</script>
3. Google Login Component: Create a component for the Google login button.
Google 로그인 구성요소: Google 로그인 버튼용 구성요소를 만듭니다.
components/GoogleLogin.vue
<template>
<v-btn color="red" @click="googleLogin">
<v-icon left>mdi-google</v-icon>
Sign in with Google
</v-btn>
</template>
<script>
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions('auth', ['googleLogin'])
}
};
</script>
Summary 요약
Using auth.onAuthStateChanged() ensures that your application always knows the current authentication state of the user and can react appropriately, whether the user signs in, signs out, or their session expires. This is essential for building robust authentication flows in modern web applications.
auth.onAuthStateChanged()를 사용하면 애플리케이션이 항상 사용자의 현재 인증 상태를 알고 사용자가 로그인하거나 로그아웃하거나 세션이 만료되는 경우 적절하게 대응할 수 있습니다. 이는 최신 웹 애플리케이션에서 강력한 인증 흐름을 구축하는 데 필수적입니다.
ChatGPT가 시키는 대로 했습니다.
1. 프로젝트 설정
vue create auto-login-2
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, PWA, Router, Vuex
? Choose a version of Vue.js that you want to start the project with 2.x
? Use history mode for router? (Requires proper server setup for index fallback in production) Yes
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated config files
? Save this as a preset for future projects? (y/N) n
2. vuetify 설치
cd auto-login-2
vue add vuetify
? Choose a preset: (Use arrow keys)
Vuetify 2 - Configure Vue CLI (advanced)
> Vuetify 2 - Vue CLI (recommended)
Vuetify 2 - Prototype (rapid development)
Vuetify 3 - Vite (preview)
Vuetify 3 - Vue CLI (preview)
3. Firebase 설정
npm install firebase
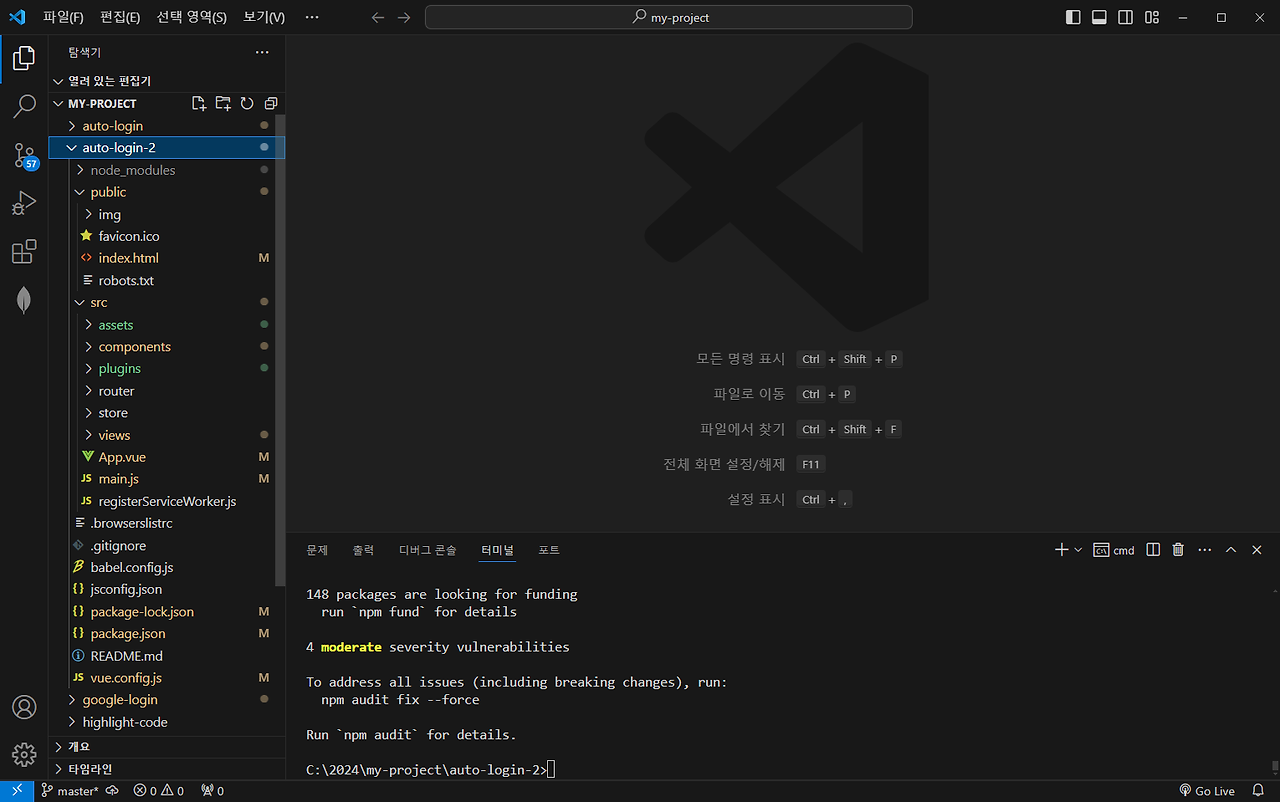
4. Firebase 초기화:
firebase.js
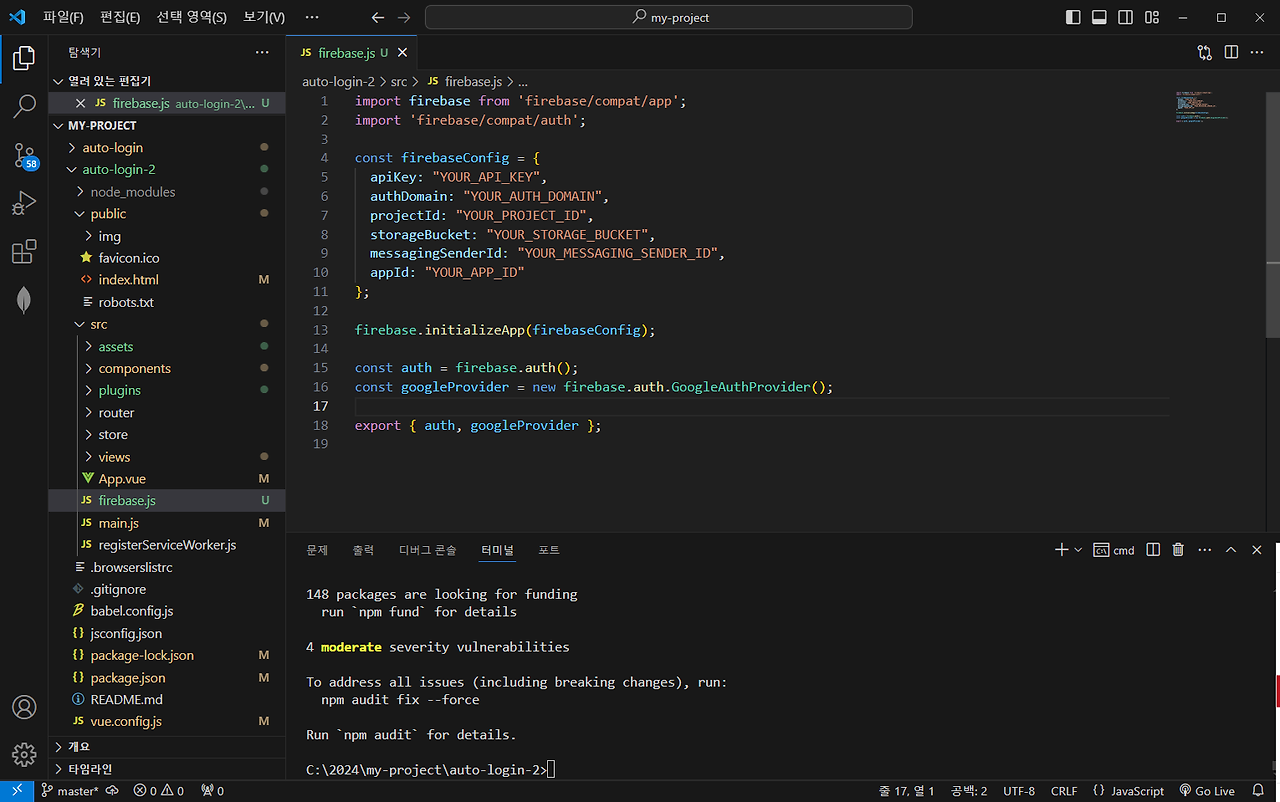
5. Vuex 스토어 모듈
store/modules/auth.js
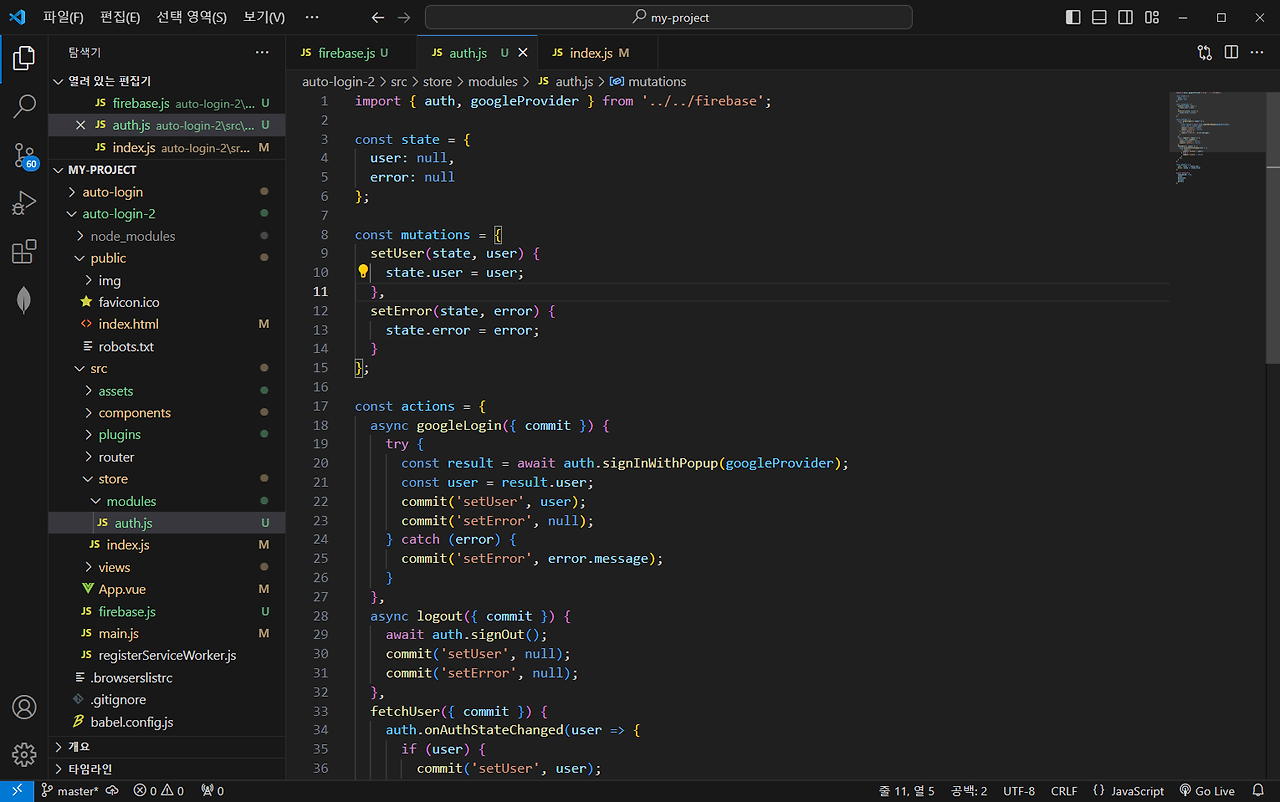
store/index.js
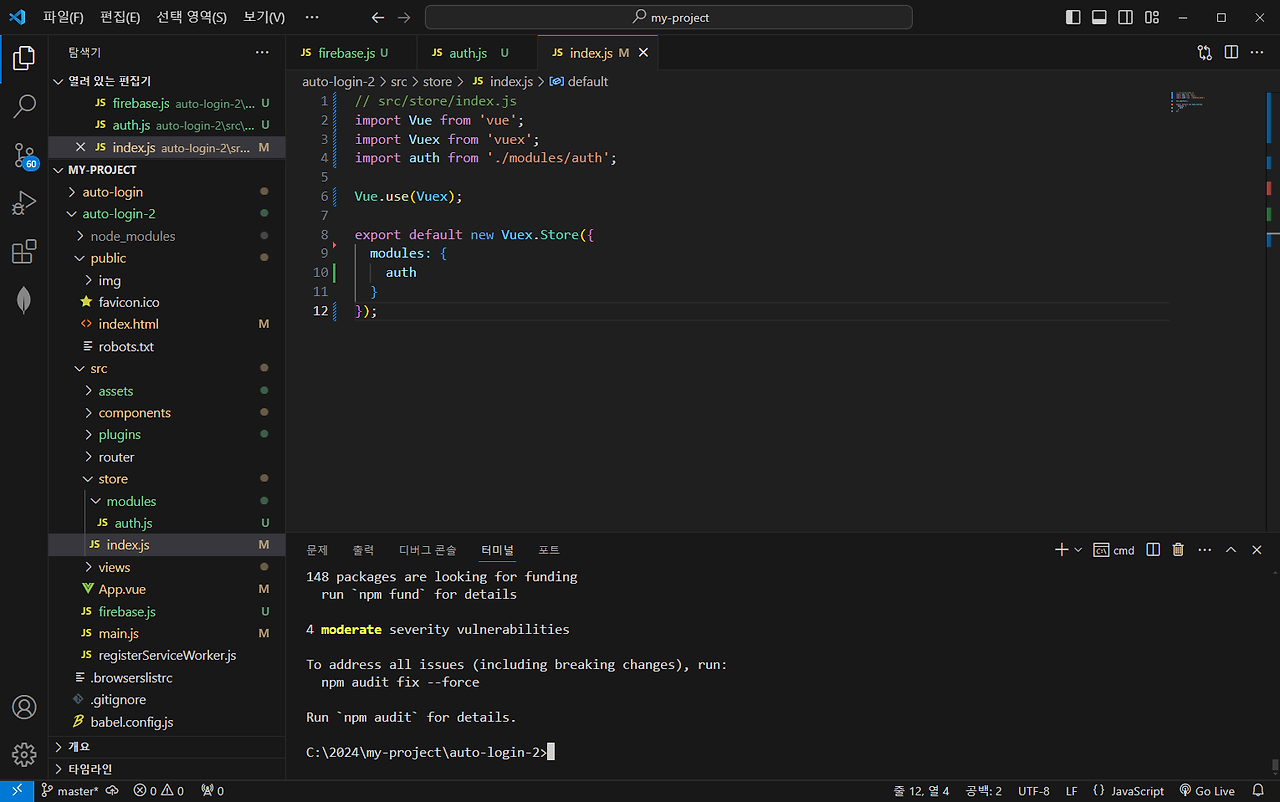
6. Google Login Component:
components/GoogleLogin.vue
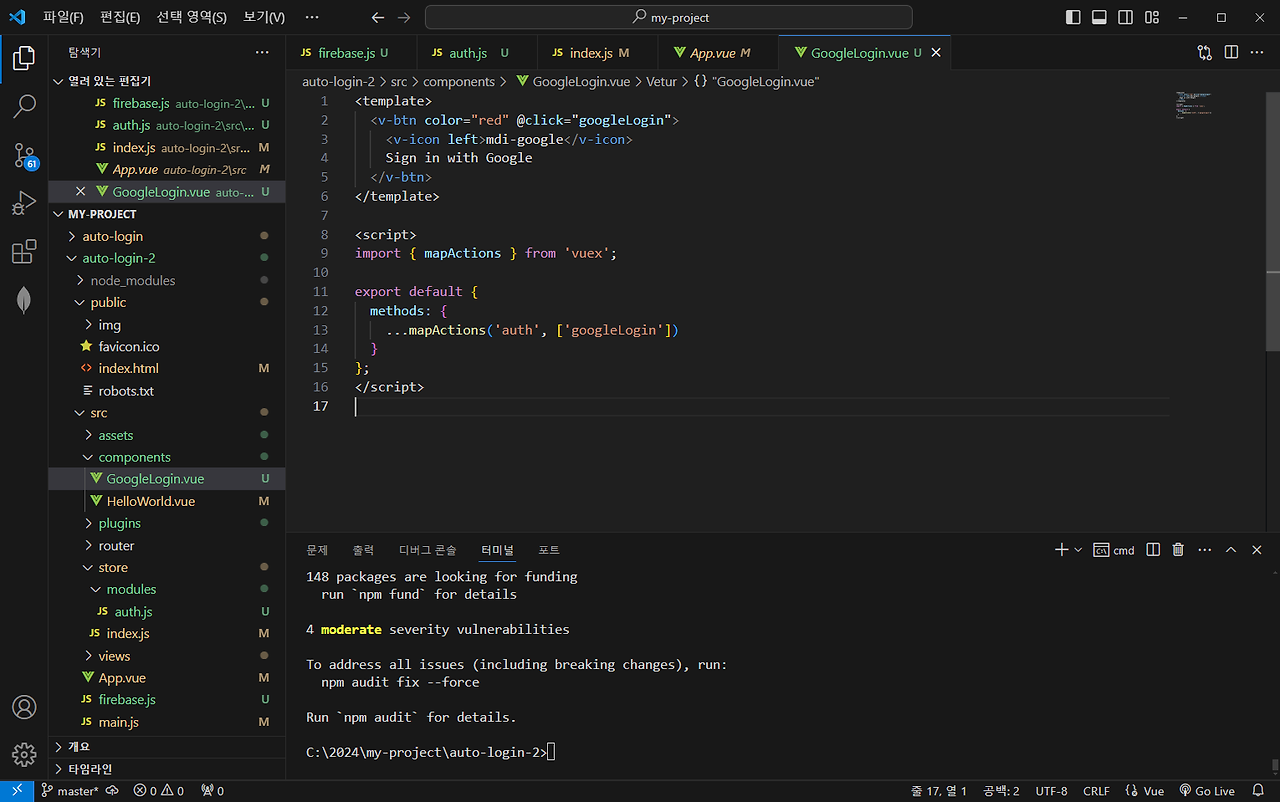
7. Main App Component
App.vue
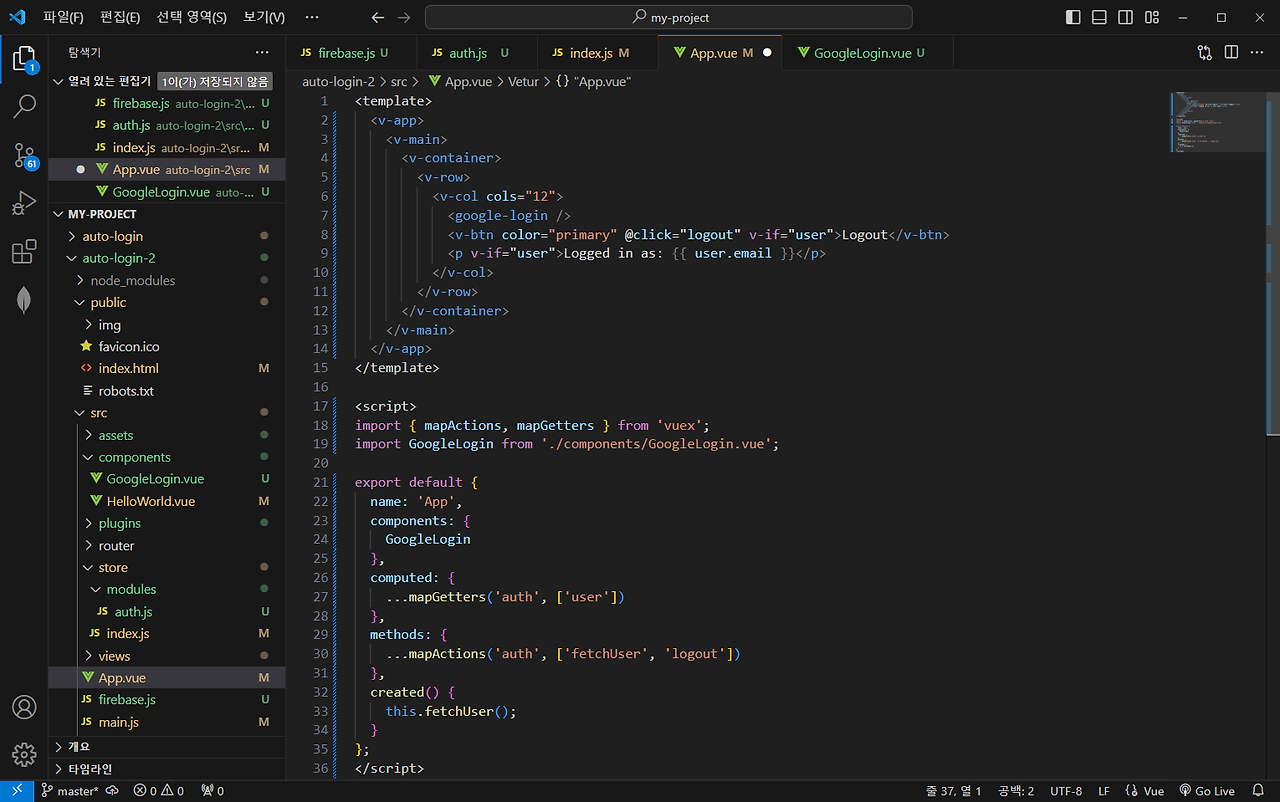
8. 실행
npm run serve
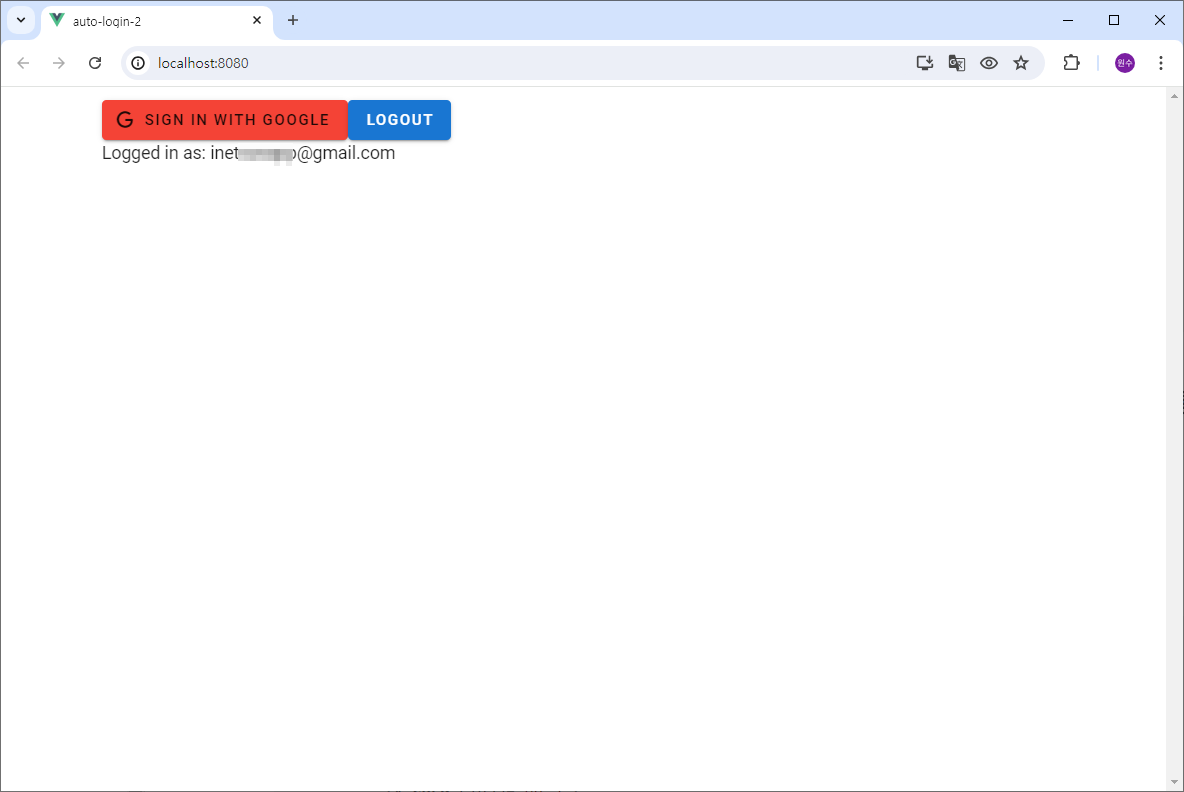
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. Firestore CRUD 디버깅 (0) | 2024.07.27 |
---|---|
ChatGPT에게 물었습니다. Firestore CRUD (0) | 2024.07.27 |
ChatGPT에게 물었습니다. 자동 로그인 (0) | 2024.07.27 |
ChatGPT에게 물었습니다. Google Login (1) | 2024.07.25 |
ChatGPT에게 물었습니다. firebase login 개선 (0) | 2024.07.25 |