30. Google Login
Show an example of google login during firebase login.
firebase login 중 google 로그인 예제 보여줘.
ChatGPT
To implement Google Login using Firebase in a Vue.js application, you need to set up Firebase Authentication and integrate it with your Vuex store and Vue components. Below is a step-by-step guide to achieve this:
Vue.js 애플리케이션에서 Firebase를 사용하여 Google 로그인을 구현하려면 Firebase 인증을 설정하고 이를 Vuex 스토어 및 Vue 구성 요소와 통합해야 합니다. 다음은 이를 달성하기 위한 단계별 가이드입니다.
Step 1: Set Up Firebase Authentication for Google Login
Google 로그인을 위한 Firebase 인증 설정
- Go to your Firebase console.
Firebase 콘솔로 이동합니다. - Navigate to the "Authentication" section.
인증' 섹션으로 이동합니다. - Under the "Sign-in method" tab, enable the "Google" provider.
"로그인 방법" 탭에서 "Google" 공급자를 활성화합니다. - Follow the instructions to set up your OAuth credentials.
지침에 따라 OAuth 자격 증명을 설정합니다.
Step 2: Update Firebase Initialization
Firebase 초기화 업데이트
Ensure that your Firebase initialization includes the Google Auth provider.
Firebase 초기화에 Google 인증 제공업체가 포함되어 있는지 확인하세요.
src/firebase.js
// src/firebase.js
import firebase from 'firebase/compat/app';
import 'firebase/compat/auth';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
firebase.initializeApp(firebaseConfig);
const auth = firebase.auth();
const googleProvider = new firebase.auth.GoogleAuthProvider();
export { auth, googleProvider };
Step 3: Update Vuex Store for Google Login
Google 로그인용 Vuex 스토어 업데이트
Add an action to your Vuex store to handle Google sign-in.
Google 로그인을 처리하기 위해 Vuex 스토어에 작업을 추가하세요.
src/store/modules/auth.js
// src/store/modules/auth.js
import { auth, googleProvider } from '../../firebase';
const state = {
user: null,
error: null
};
const mutations = {
setUser(state, user) {
state.user = user;
},
setError(state, error) {
state.error = error;
}
};
const actions = {
async login({ commit }, { email, password }) {
try {
const { user } = await auth.signInWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async register({ commit }, { email, password }) {
try {
const { user } = await auth.createUserWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async googleLogin({ commit }) {
try {
const { user } = await auth.signInWithPopup(googleProvider);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async logout({ commit }) {
await auth.signOut();
commit('setUser', null);
commit('setError', null);
}
};
const getters = {
user: state => state.user,
error: state => state.error
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
Step 4: Create Google Login Button Component
Google 로그인 버튼 구성요소 만들기
Create a component for the Google login button.
Google 로그인 버튼에 대한 구성요소를 만듭니다.
src/components/GoogleLogin.vue
<!-- src/components/GoogleLogin.vue -->
<template>
<v-btn color="red" @click="googleLogin">
<v-icon left>mdi-google</v-icon>
Sign in with Google
</v-btn>
</template>
<script>
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions('auth', ['googleLogin'])
}
};
</script>
Step 5: Update the Main App Component
기본 앱 구성요소 업데이트
Include the GoogleLogin component in your main app component or wherever needed.
기본 앱 구성 요소 또는 필요한 곳에 GoogleLogin 구성 요소를 포함하세요.
src/App.vue
<!-- src/App.vue -->
<template>
<v-app>
<v-main>
<v-container>
<v-row>
<v-col cols="12" sm="6">
<login />
</v-col>
<v-col cols="12" sm="6">
<register />
</v-col>
</v-row>
<v-row>
<v-col cols="12">
<user-info />
</v-col>
</v-row>
<v-row>
<v-col cols="12">
<google-login />
</v-col>
</v-row>
</v-container>
</v-main>
</v-app>
</template>
<script>
import Login from './components/Login.vue';
import Register from './components/Register.vue';
import UserInfo from './components/UserInfo.vue';
import GoogleLogin from './components/GoogleLogin.vue';
export default {
name: 'App',
components: {
Login,
Register,
UserInfo,
GoogleLogin
}
};
</script>
Step 6: Run Your Application
애플리케이션 실행
Run your application to see the login forms, registration form, and the Google login button in action.
애플리케이션을 실행하여 로그인 양식, 등록 양식 및 Google 로그인 버튼이 작동하는 모습을 확인하세요.
npm run serve
Summary
요약
By following these steps, you ensure that the user information returned by Google Login using Firebase is properly handled and displayed in your Vue.js application. This setup keeps your code organized and leverages Vuex for state management, ensuring that user information is easily accessible throughout your application.
다음 단계를 수행하면 Firebase를 사용하여 Google 로그인에서 반환된 사용자 정보가 Vue.js 애플리케이션에 올바르게 처리되고 표시되는지 확인할 수 있습니다. 이 설정은 코드를 체계적으로 유지하고 상태 관리를 위해 Vuex를 활용하여 애플리케이션 전체에서 사용자 정보에 쉽게 액세스할 수 있도록 보장합니다.
ChatGPT가 시키는 대로 했습니다.
Google 로그인을 위한 Firebase 인증 설정
먼저 Google 로그인을 위한 Firebase 인증 설정이 되어 있어야 합니다.
- Firebase 콘솔로 이동합니다.
- 인증' 섹션으로 이동합니다.
- "로그인 방법" 탭에서 "Google" 공급자를 활성화합니다.
- 지침에 따라 OAuth 자격 증명을 설정합니다.
프로젝트 만들기
1. 프로젝트 설정
vue create my-login-1
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, PWA, Router, Vuex
? Choose a version of Vue.js that you want to start the project with 2.x
? Use history mode for router? (Requires proper server setup for index fallback in production) Yes
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated config files
? Save this as a preset for future projects? (y/N) n
2. vuetify 설치
cd my-login-1
vue add vuetify
? Choose a preset: (Use arrow keys)
Vuetify 2 - Configure Vue CLI (advanced)
> Vuetify 2 - Vue CLI (recommended)
Vuetify 2 - Prototype (rapid development)
Vuetify 3 - Vite (preview)
Vuetify 3 - Vue CLI (preview)
3. Firebase 설정
npm install firebase
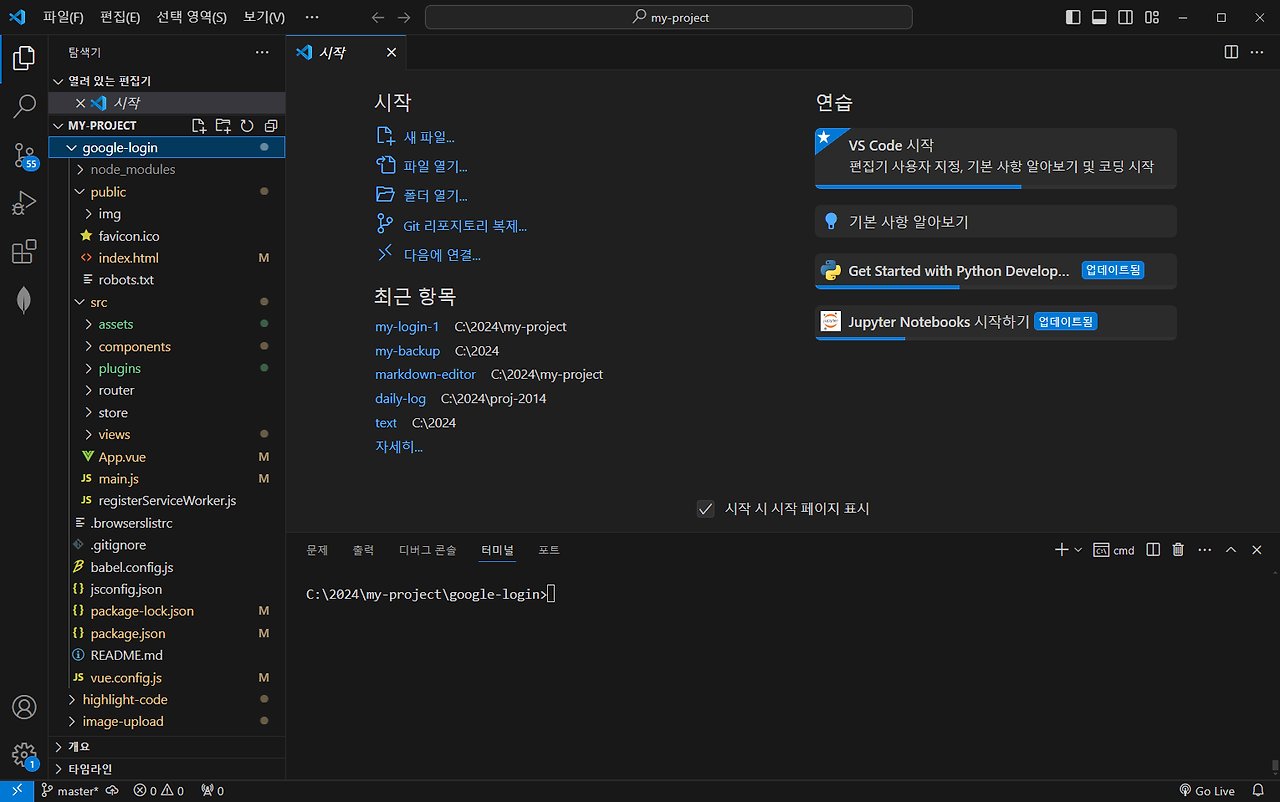
4. Firebase를 초기화
src/firebase.js
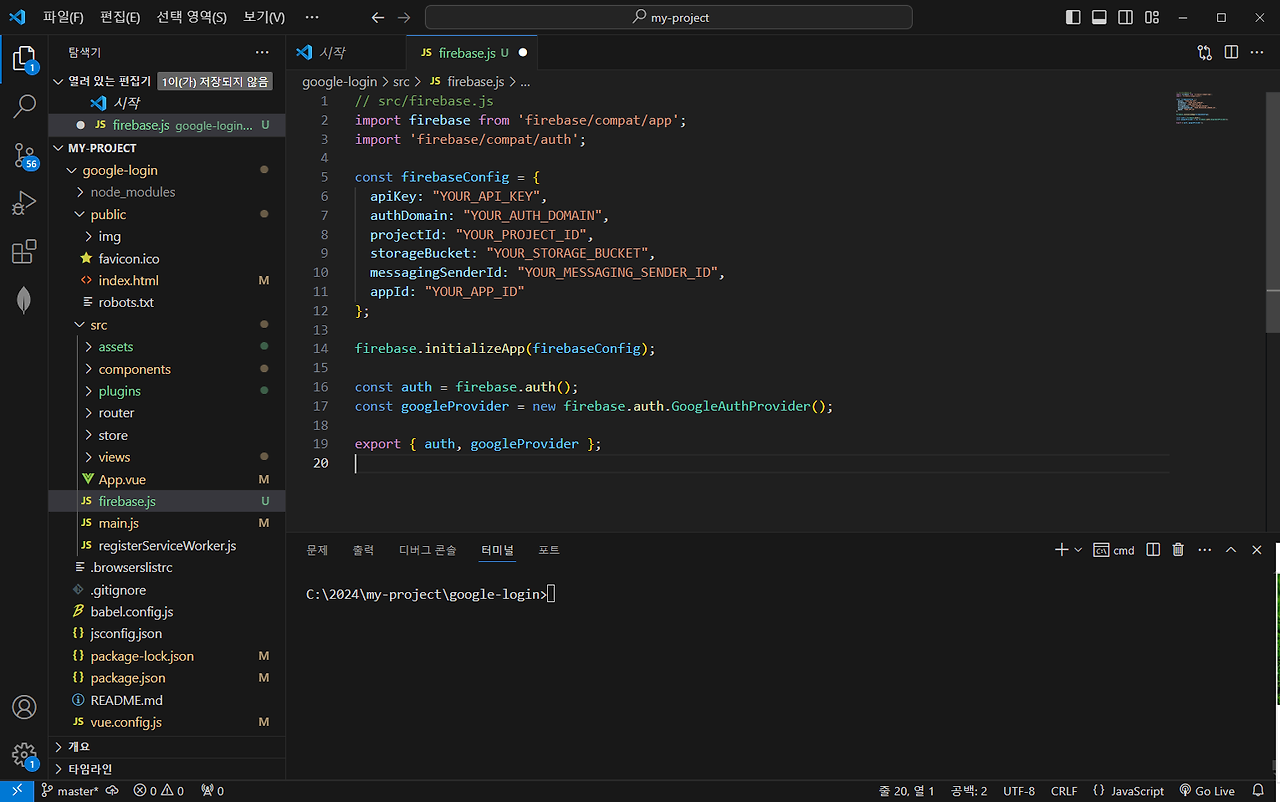
5. Google 로그인용 Vuex 스토어 업데이트
src/store/modules/auth.js
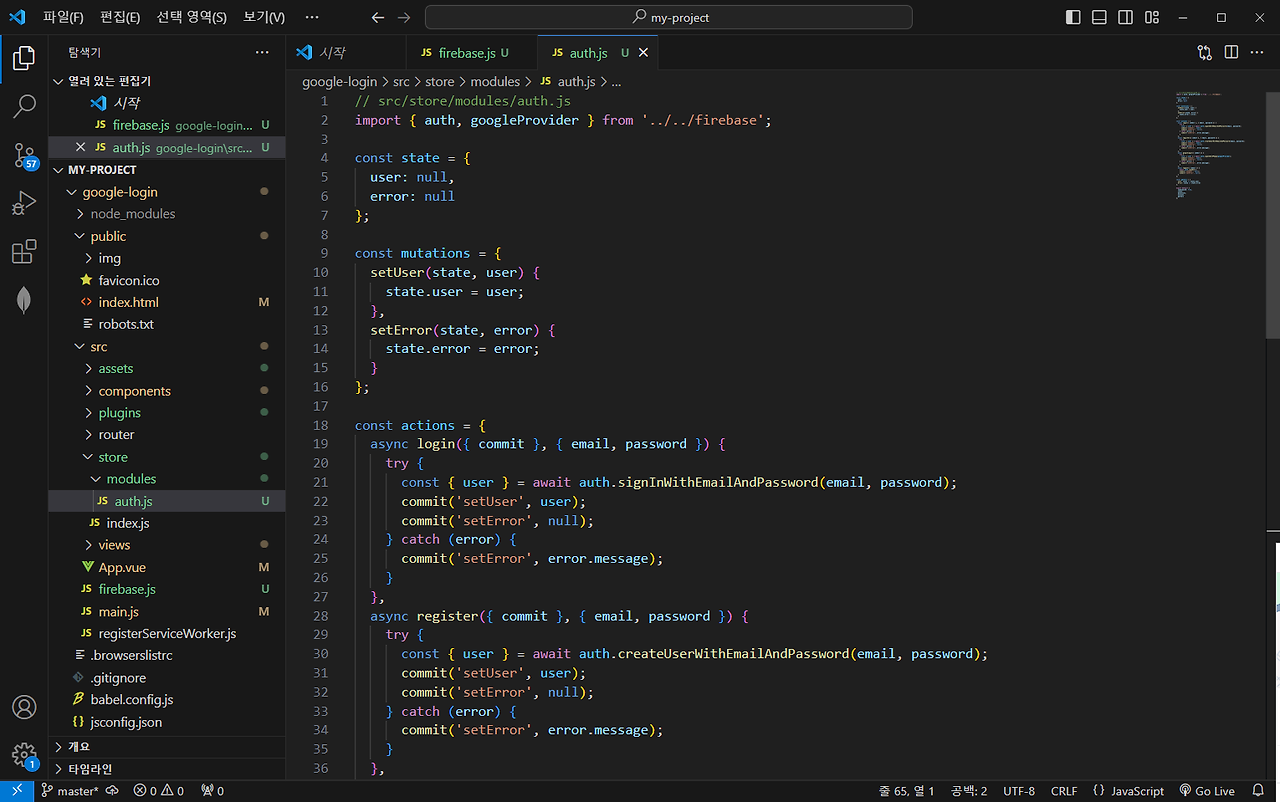
src/store/index.js
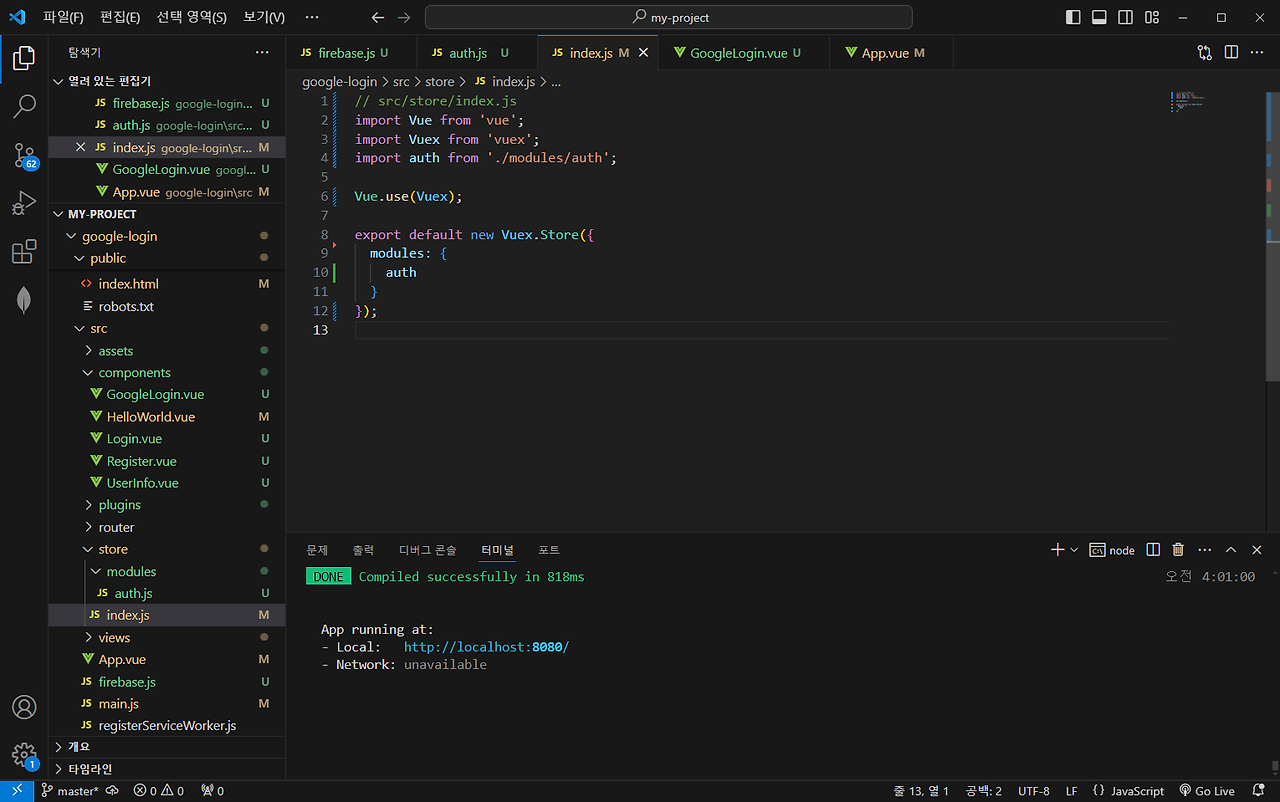
6. Google 로그인 버튼 구성요소 만들기
src/components/GoogleLogin.vue
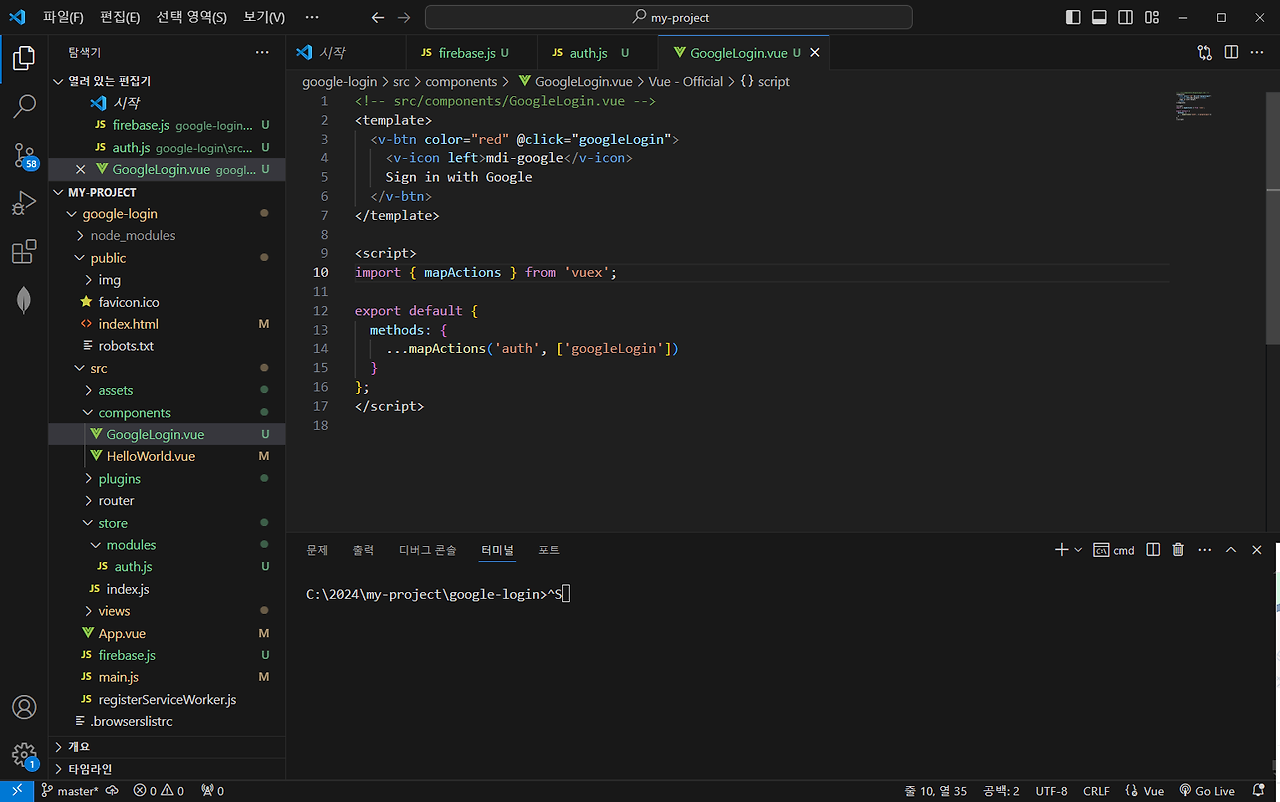
7. Register.vue, UserInfo.vue, Login.vue
my-login-1 프로젝트에서 만든 것으로 가져옵니다. 이 프로젝트는 사실 my-login-1를 고도화하는 것입니다.
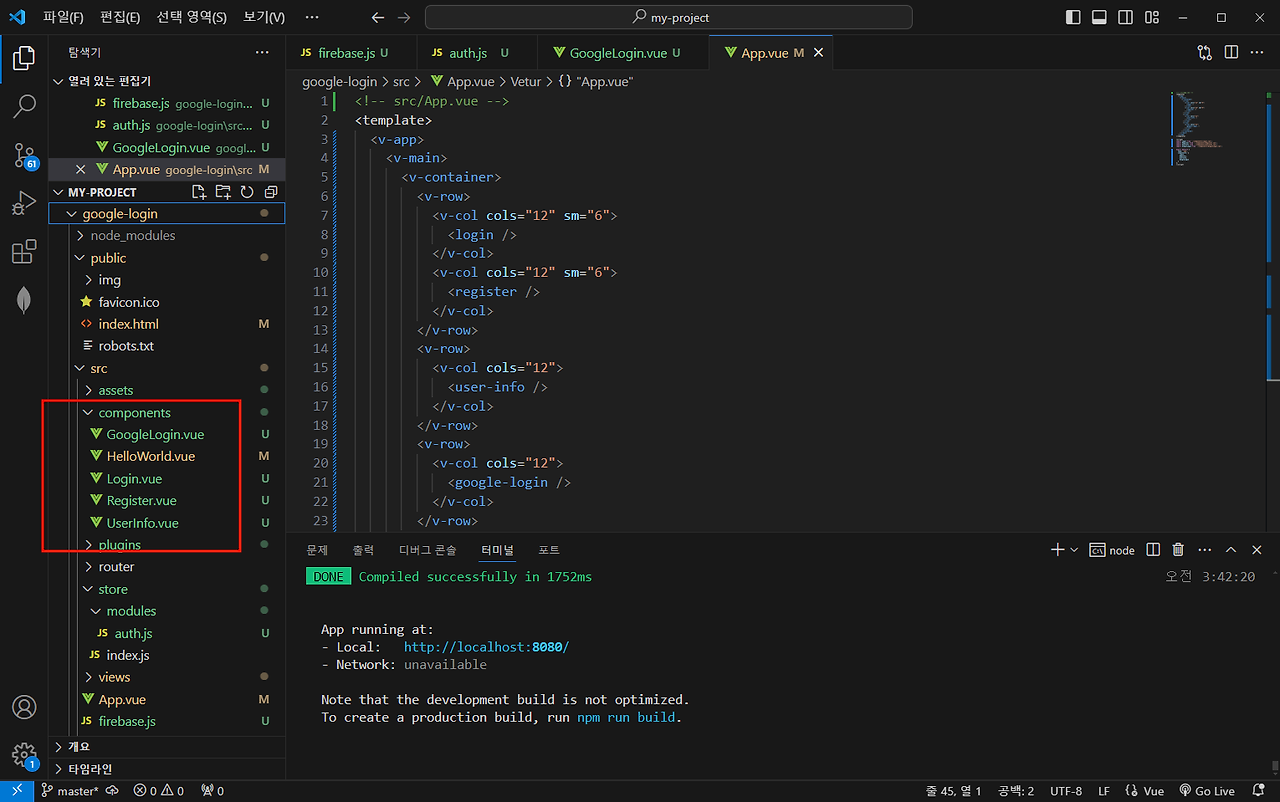
7. 기본 앱 구성요소 업데이트
src/App.vue
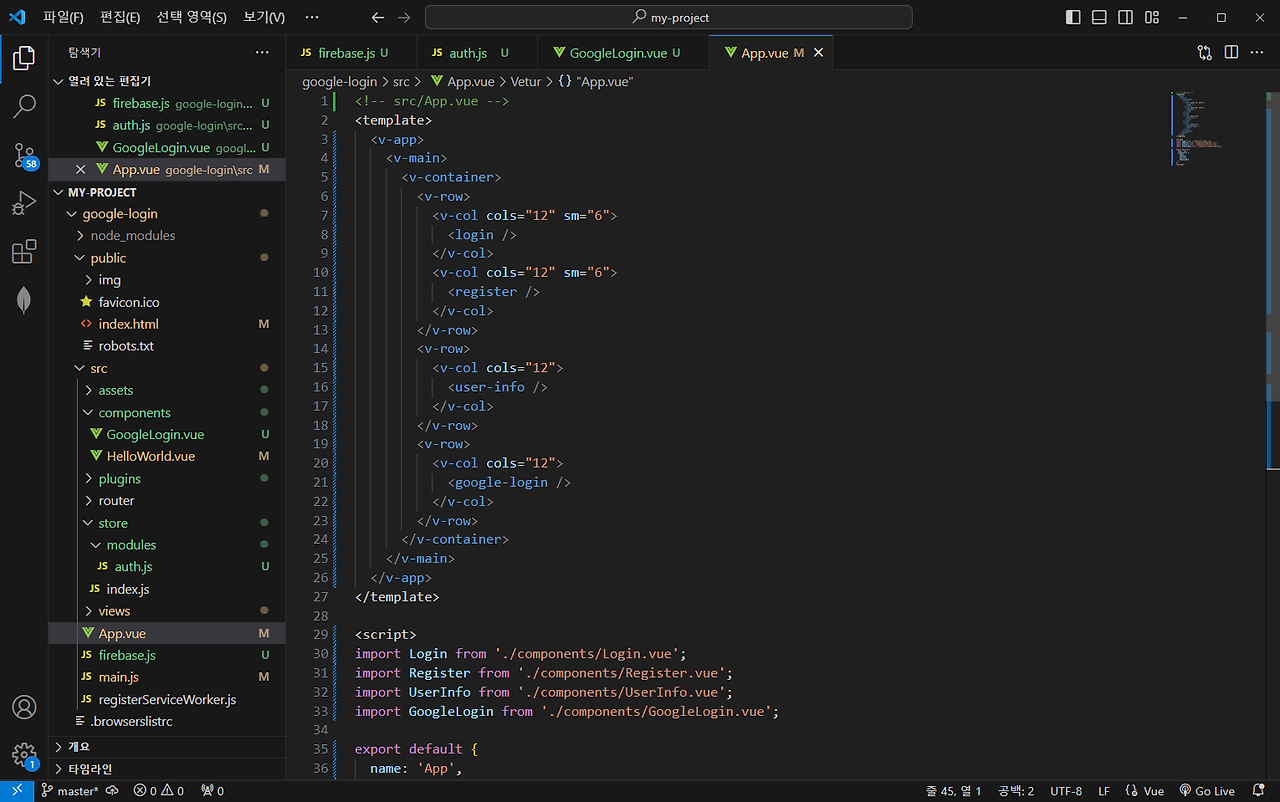
8. 애플리케이션 실행
npm run serve
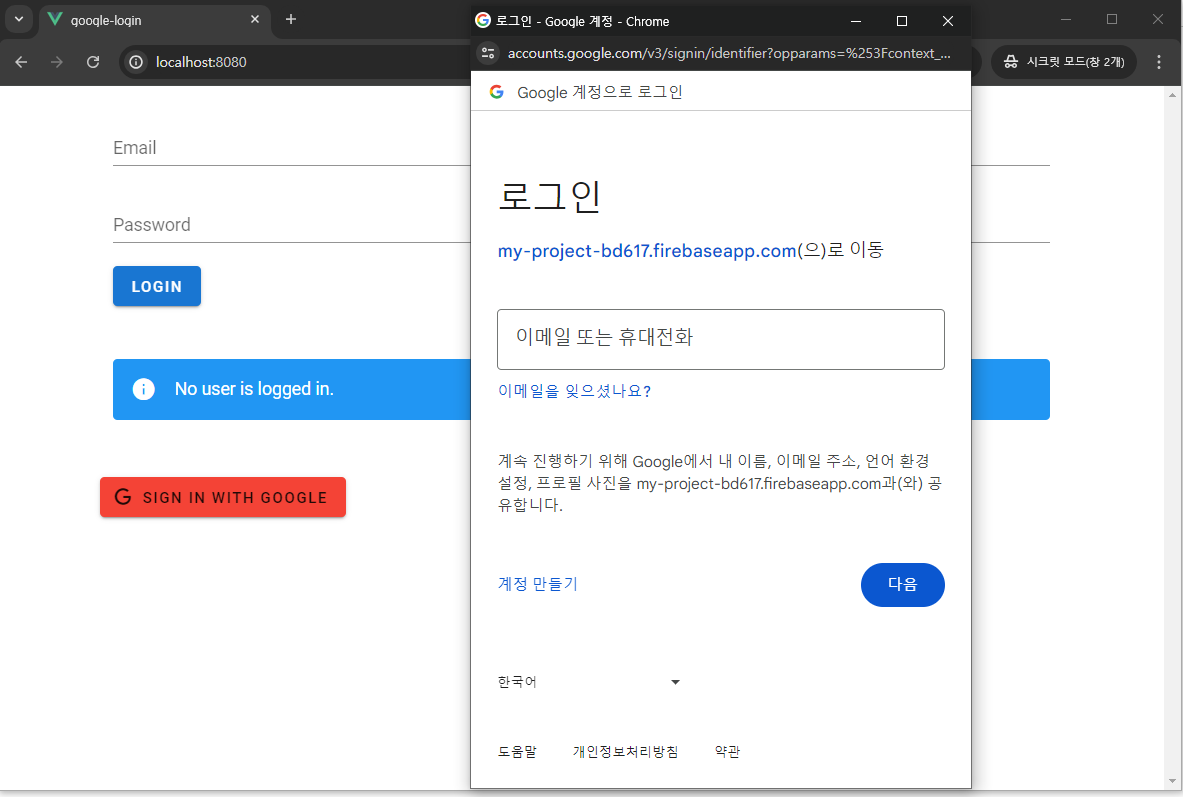
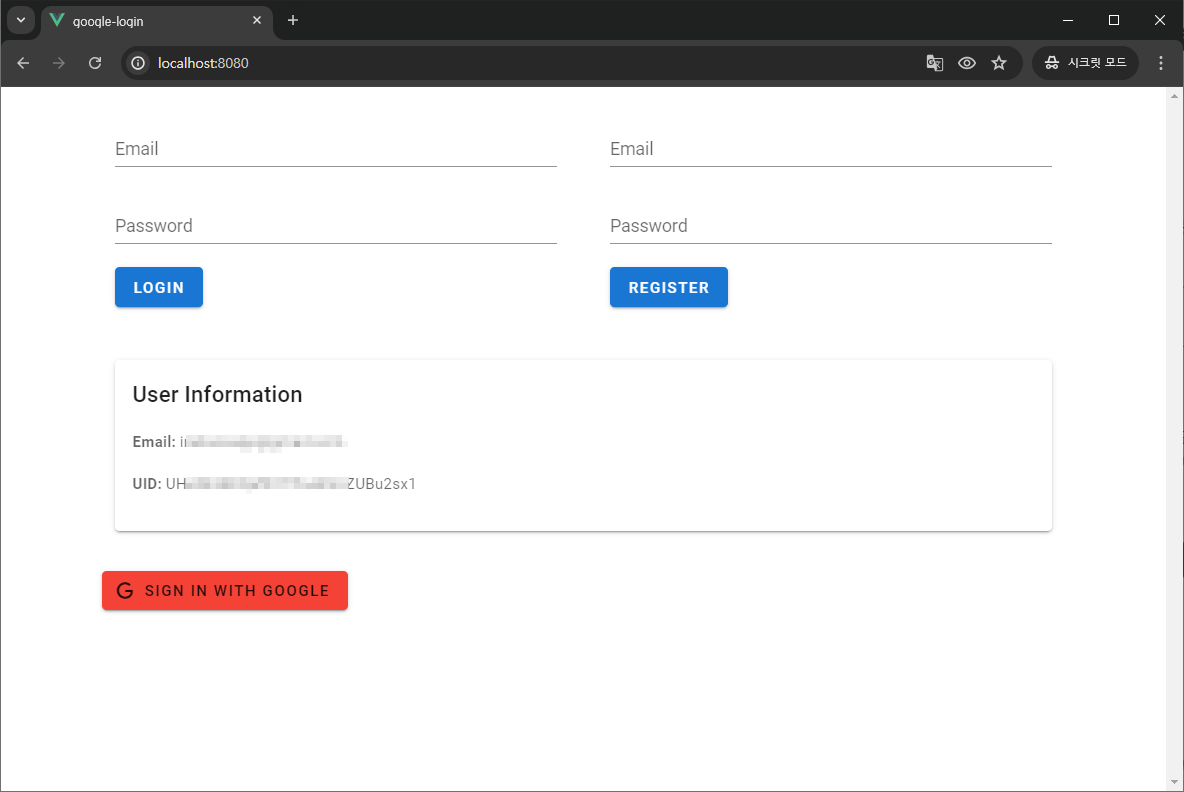
9. 소스
src/store/modules/auth.js
// src/store/modules/auth.js
import { auth, googleProvider } from '../../firebase';
const state = {
user: null,
error: null
};
const mutations = {
setUser(state, user) {
state.user = user;
},
setError(state, error) {
state.error = error;
}
};
const actions = {
async login({ commit }, { email, password }) {
try {
const { user } = await auth.signInWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async register({ commit }, { email, password }) {
try {
const { user } = await auth.createUserWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async googleLogin({ commit }) {
try {
const result = await auth.signInWithPopup(googleProvider);
const user = result.user;
console.log('Google Sign-In successful', user);
commit('setUser', user);
commit('setError', null);
} catch (error) {
console.error('Google Sign-In error', error);
commit('setError', error.message);
}s
},
async logout({ commit }) {
await auth.signOut();
commit('setUser', null);
commit('setError', null);
}
};
const getters = {
user: state => state.user,
error: state => state.error
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
src/store/index.js
// src/store/index.js
import Vue from 'vue';
import Vuex from 'vuex';
import auth from './modules/auth';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
auth
}
});
src/components/GoogleLogin.vue
<!-- src/components/GoogleLogin.vue -->
<template>
<v-btn color="red" @click="googleLogin">
<v-icon left>mdi-google</v-icon>
Sign in with Google
</v-btn>
</template>
<script>
import { mapActions } from 'vuex';
export default {
methods: {
...mapActions('auth', ['googleLogin'])
}
};
</script>
src/components/Login.vue
<!-- src/components/Login.vue -->
<template>
<v-container>
<v-form @submit.prevent="loginUser">
<v-text-field v-model="email" label="Email" type="email" required></v-text-field>
<v-text-field v-model="password" label="Password" type="password" required></v-text-field>
<v-btn type="submit" color="primary">Login</v-btn>
<v-alert v-if="error" type="error">{{ error }}</v-alert>
</v-form>
</v-container>
</template>
<script>
import { mapActions, mapGetters } from 'vuex';
export default {
data() {
return {
email: '',
password: ''
};
},
computed: {
...mapGetters('auth', ['error'])
},
methods: {
...mapActions('auth', ['login']),
async loginUser() {
await this.login({ email: this.email, password: this.password });
}
}
};
</script>
src/components/Register.vue
<!-- src/components/Register.vue -->
<template>
<v-container>
<v-form @submit.prevent="userRegister">
<v-text-field v-model="email" label="Email" type="email" required></v-text-field>
<v-text-field v-model="password" label="Password" type="password" required></v-text-field>
<v-btn type="submit" color="primary">Register</v-btn>
<v-alert v-if="error" type="error">{{ error }}</v-alert>
</v-form>
</v-container>
</template>
<script>
import { mapActions, mapGetters } from 'vuex';
export default {
data() {
return {
email: '',
password: ''
};
},
computed: {
...mapGetters('auth', ['error'])
},
methods: {
...mapActions('auth', ['register']),
async userRegister() {
await this.register({ email: this.email, password: this.password });
}
}
};
</script>
src/components/UserInfo.vue
<!-- src/components/UserInfo.vue -->
<template>
<v-container>
<v-card v-if="user">
<v-card-title>User Information</v-card-title>
<v-card-text>
<p><strong>Email:</strong> {{ user.email }}</p>
<p><strong>UID:</strong> {{ user.uid }}</p>
</v-card-text>
</v-card>
<v-alert v-else type="info">No user is logged in.</v-alert>
</v-container>
</template>
<script>
import { mapGetters } from 'vuex';
export default {
computed: {
...mapGetters('auth', ['user'])
}
};
</script>
src/App.vue
<!-- src/App.vue -->
<template>
<v-app>
<v-main>
<v-container>
<v-row>
<v-col cols="12" sm="6">
<login />
</v-col>
<v-col cols="12" sm="6">
<register />
</v-col>
</v-row>
<v-row>
<v-col cols="12">
<user-info />
</v-col>
</v-row>
<v-row>
<v-col cols="12">
<google-login />
</v-col>
</v-row>
</v-container>
</v-main>
</v-app>
</template>
<script>
import Login from './components/Login.vue';
import Register from './components/Register.vue';
import UserInfo from './components/UserInfo.vue';
import GoogleLogin from './components/GoogleLogin.vue';
export default {
name: 'App',
components: {
Login,
Register,
UserInfo,
GoogleLogin
}
};
</script>
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. onAuthStateChanged (0) | 2024.07.27 |
---|---|
ChatGPT에게 물었습니다. 자동 로그인 (0) | 2024.07.27 |
ChatGPT에게 물었습니다. firebase login 개선 (0) | 2024.07.25 |
ChatGPT가 시키는 대로 했습니다. firebase login - 디버깅 (0) | 2024.07.25 |
ChatGPT가 시키는 대로 했습니다. firebase login - Example (0) | 2024.07.25 |