29. ChatGPT에게 물었습니다.
firebase 로그인으로 로그인한 후 사용자 정보를 화면에 표시하고 싶어.
After logging in with firebase login, I want to display the user info returned on the screen.
ChatGPT
To display the user info returned by Firebase after logging in, you need to ensure that the user data is properly stored in the Vuex store and then access this data in your Vue components. 로그인 후 Firebase에서 반환한 사용자 정보를 표시하려면 사용자 데이터가 Vuex 저장소에 제대로 저장되어 있는지 확인한 다음 Vue 구성 요소에서 이 데이터에 액세스해야 합니다.
Here's a step-by-step guide to achieve this:
이를 달성하기 위한 단계별 가이드는 다음과 같습니다.
Step 1: Modify Vuex Store to Handle User Info : 사용자 정보를 처리하도록 Vuex Store 수정
Ensure that your Vuex store's auth module properly stores the user info.
Vuex 스토어의 'auth' 모듈이 사용자 정보를 올바르게 저장하는지 확인하세요.
src/store/modules/auth.js
// src/store/modules/auth.js
import { auth } from '../../firebase';
const state = {
user: null,
error: null
};
const mutations = {
setUser(state, user) {
state.user = user;
},
setError(state, error) {
state.error = error;
}
};
const actions = {
async login({ commit }, { email, password }) {
try {
const { user } = await auth.signInWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async register({ commit }, { email, password }) {
try {
const { user } = await auth.createUserWithEmailAndPassword(email, password);
commit('setUser', user);
commit('setError', null);
} catch (error) {
commit('setError', error.message);
}
},
async logout({ commit }) {
await auth.signOut();
commit('setUser', null);
commit('setError', null);
}
};
const getters = {
user: state => state.user,
error: state => state.error
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
Step 2: Create a Component to Display User Info : 사용자 정보를 표시하는 구성 요소 만들기
Create a component that will display the logged-in user's information.
로그인한 사용자의 정보를 표시하는 구성 요소를 만듭니다.
src/components/UserInfo.vue
<!-- src/components/UserInfo.vue -->
<template>
<v-container>
<v-card v-if="user">
<v-card-title>User Information</v-card-title>
<v-card-text>
<p><strong>Email:</strong> {{ user.email }}</p>
<p><strong>UID:</strong> {{ user.uid }}</p>
</v-card-text>
</v-card>
<v-alert v-else type="info">No user is logged in.</v-alert>
</v-container>
</template>
<script>
import { mapGetters } from 'vuex';
export default {
computed: {
...mapGetters('auth', ['user'])
}
};
</script>
<style scoped>
/* Optional styles */
</style>
Step 3: Update the Main App Component : 기본 앱 구성요소 업데이트
Include the UserInfo component in your main app component or where you want to display the user information.
기본 앱 구성 요소 또는 사용자 정보를 표시하려는 위치에 UserInfo 구성 요소를 포함하세요.
src/App.vue
<!-- src/App.vue -->
<template>
<v-app>
<v-main>
<v-container>
<v-row>
<v-col cols="12" sm="6">
<login />
</v-col>
<v-col cols="12" sm="6">
<register />
</v-col>
</v-row>
<v-row>
<v-col cols="12">
<user-info />
</v-col>
</v-row>
</v-container>
</v-main>
</v-app>
</template>
<script>
import Login from './components/Login.vue';
import Register from './components/Register.vue';
import UserInfo from './components/UserInfo.vue';
export default {
name: 'App',
components: {
Login,
Register,
UserInfo
}
};
</script>
<style>
@import '~vuetify/dist/vuetify.min.css';
</style>
Step 4: Run Your Application : 애플리케이션 실행
Run your application to see the login form, registration form, and the user information displayed upon successful login.
애플리케이션을 실행하여 로그인 양식, 등록 양식, 로그인 성공 시 표시되는 사용자 정보를 확인하세요.
npm run serve
Summary 요약
By following these steps, you ensure that the user information returned by Firebase is stored in the Vuex store and properly accessed and displayed in your Vue components. This approach keeps your code organized and leverages Vuex for state management, ensuring that user information is easily accessible throughout your application.
다음 단계를 수행하면 Firebase에서 반환된 사용자 정보가 Vuex 저장소에 저장되고 Vue 구성 요소에 올바르게 액세스되어 표시되는지 확인할 수 있습니다. 이 접근 방식은 코드를 체계적으로 유지하고 상태 관리를 위해 Vuex를 활용하여 애플리케이션 전체에서 사용자 정보에 쉽게 액세스할 수 있도록 보장합니다.
ChatGPT가 시키는 대로 수정하였습니다.
my-login-1 프로젝트 설정은 여기를 참조하세요.
Step 1: Modify Vuex Store to Handle User Info : 사용자 정보를 처리하도록 Vuex Store 수정
src/store/modules/auth.js 소스 코드의 변경 사항이 없습니다.
Step 2: Create a Component to Display User Info : 사용자 정보를 표시하는 구성 요소 만들기
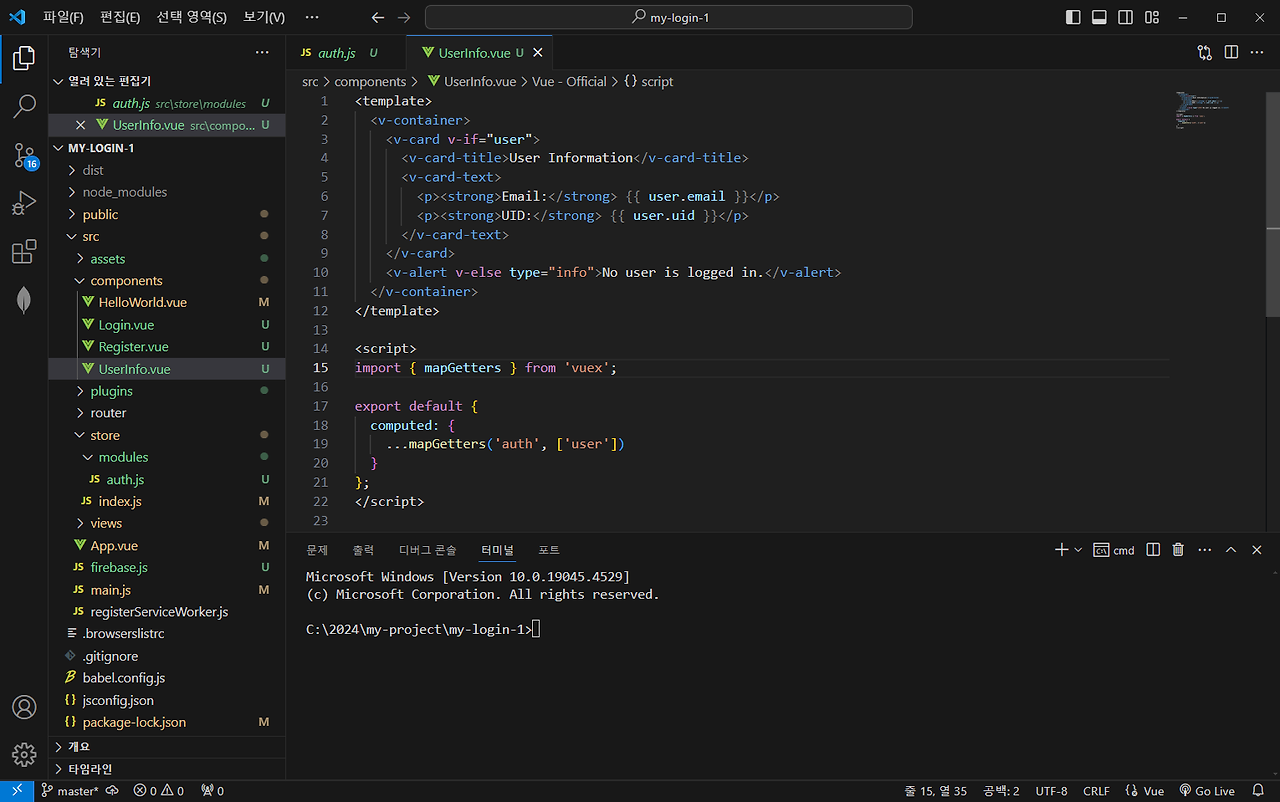
Step 3: Update the Main App Component : 기본 앱 구성요소 업데이트
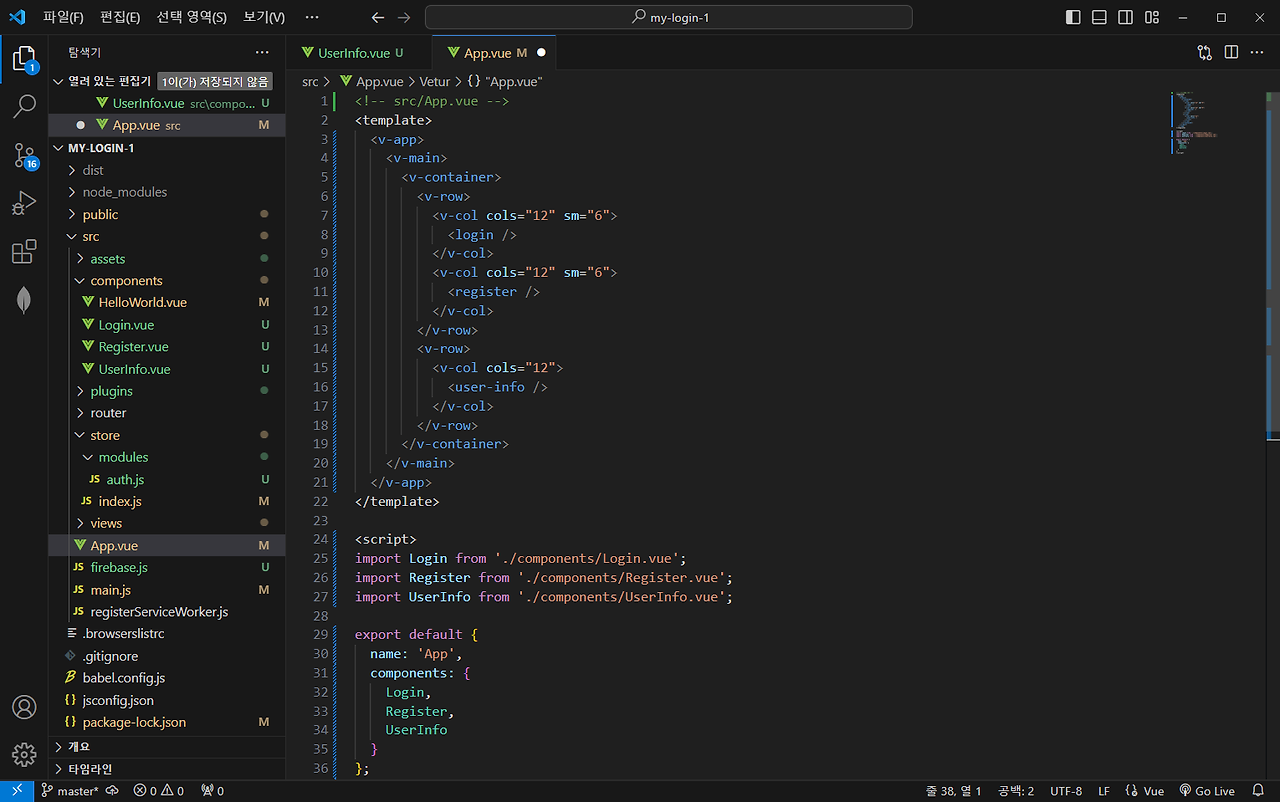
Step 4: Run Your Application : 애플리케이션 실행
npm run serve
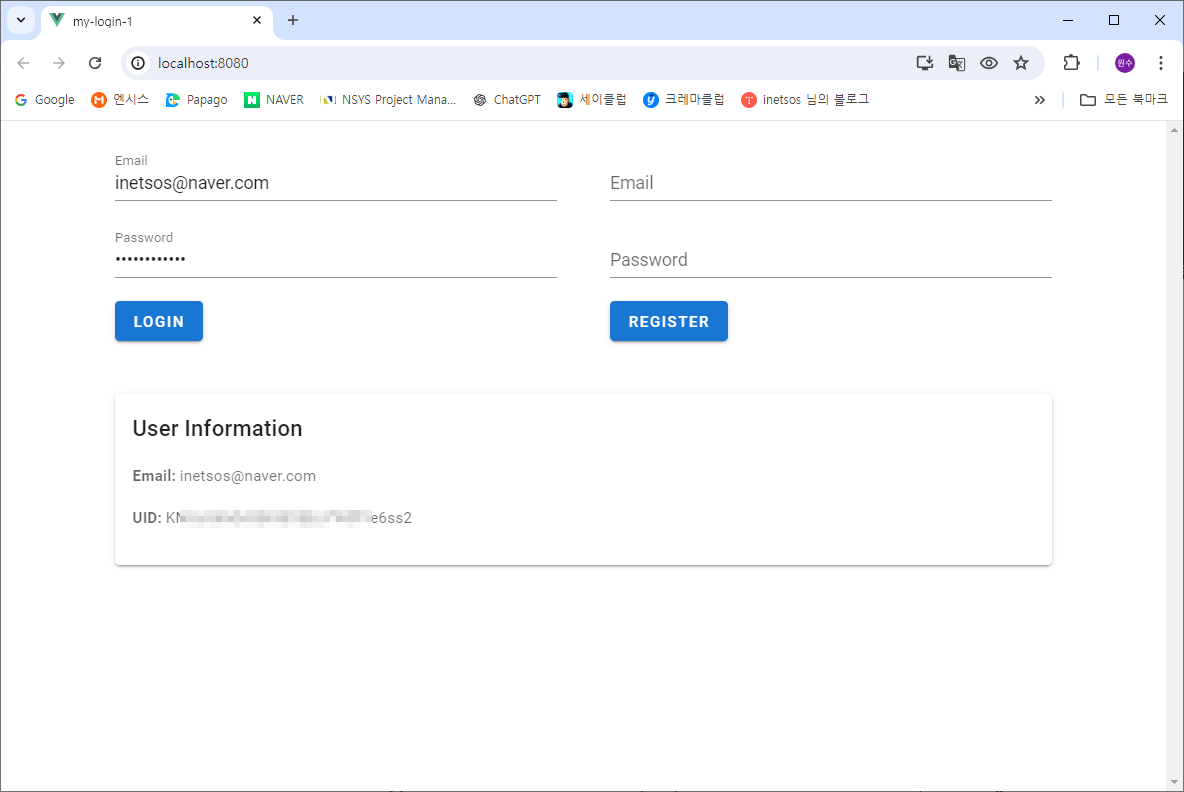
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. 자동 로그인 (0) | 2024.07.27 |
---|---|
ChatGPT에게 물었습니다. Google Login (1) | 2024.07.25 |
ChatGPT가 시키는 대로 했습니다. firebase login - 디버깅 (0) | 2024.07.25 |
ChatGPT가 시키는 대로 했습니다. firebase login - Example (0) | 2024.07.25 |
ChatGPT에게 물었습니다. firebase login 1 (0) | 2024.07.25 |