계정 정보를 입력받아 구글에 계정 등록을 하고, 구글 계정의 uid와 함께 mylog 웹앱에도 계정 정보를 저장하게 됩니다. 사용자 인증 관련은 구글 계정을 사용하고, mylog에 저장되는 계정 정보는 각종 앱 운영에 필요할 때 사용하게 됩니다. 그러므로 사용자의 비밀번호는 mylog 계정에 저장되지 않습니다.
- UI를 만들기
- 계정 정보를 입력 받아 구글에 계정 등록하기
- 구글 계정의 uid와 계정 정보를 mylog 계정 DB에 저장하기
계정 만들기를 진행하면서 firebase 설정을 하고, 계정 정보를 firestore DB에 저장을 하고, 입력 중 각종 오류에 대해 알림을 나타내는 기능 등을 구현하게 됩니다.
계정 등록을 위한 화면부터 만들어 봅시다.
ChatGPT에게 물었습니다.
Create an account registration screen using vuetify.
vuetify를 사용하여 계정 등록 화면을 만들어줘.
ChatGPT
To create an account registration screen using Vuetify in a Vue.js project, we'll build a form with fields for username, email, password, and password confirmation. We will also add some basic validation rules for each input.
Vue.js 프로젝트에서 Vuetify를 사용하여 계정 등록 화면을 생성하기 위해 사용자 이름, 이메일, 비밀번호 및 비밀번호 확인을 위한 필드가 있는 양식을 작성하겠습니다. 또한 각 입력에 대해 몇 가지 기본 유효성 검사 규칙을 추가할 것입니다.
1. Set Up a Vue.js Project with Vuetify
1. Vuetify를 사용하여 Vue.js 프로젝트 설정
If you haven’t set up a Vue.js project with Vuetify, you can follow these steps. Otherwise, skip to step 2.
Vuetify로 Vue.js 프로젝트를 설정하지 않은 경우 다음 단계를 따르세요. 그렇지 않으면 2단계로 건너뜁니다.
# Install Vue CLI globally if you haven't already
npm install -g @vue/cli
# Create a new Vue project
vue create vuetify-registration-app
# Navigate into the project directory
cd vuetify-registration-app
# Add Vuetify to your project
vue add vuetify
Choose the default Vuetify setup or customize it according to your needs during the installation process.
기본 Vuetify 설정을 선택하거나 설치 프로세스 중 필요에 따라 사용자 정의하세요.
2. Create a Registration Component
2. 등록 구성 요소 만들기
Once the project is set up with Vuetify, create a new component for the registration screen.
Vuetify로 프로젝트가 설정되면 등록 화면에 대한 새 구성 요소를 만듭니다.
Step 1: Create a Register.vue Component
1단계: Register.vue 구성 요소 만들기
In the src/components directory, create a new file named Register.vue:
src/comComponents 디렉터리에 Register.vue라는 새 파일을 만듭니다.
touch src/components/Register.vue
Step 2: Add Code to Register.vue
2단계: Register.vue에 코드 추가
Open Register.vue and add the following code to build a registration form using Vuetify components:
'Register.vue'를 열고 다음 코드를 추가하여 Vuetify 구성 요소를 사용하여 등록 양식을 작성합니다.
<template>
<v-container class="fill-height" fluid>
<v-row align="center" justify="center">
<v-col cols="12" sm="8" md="4">
<v-card>
<v-card-title class="text-h5">
Register
</v-card-title>
<v-card-text>
<v-form ref="form" v-model="valid" lazy-validation>
<v-text-field
v-model="username"
:rules="usernameRules"
label="Username"
prepend-icon="mdi-account"
required
></v-text-field>
<v-text-field
v-model="email"
:rules="emailRules"
label="Email"
prepend-icon="mdi-email"
required
></v-text-field>
<v-text-field
v-model="password"
:rules="passwordRules"
label="Password"
prepend-icon="mdi-lock"
type="password"
required
></v-text-field>
<v-text-field
v-model="confirmPassword"
:rules="confirmPasswordRules"
label="Confirm Password"
prepend-icon="mdi-lock"
type="password"
required
></v-text-field>
<v-btn
:disabled="!valid"
color="primary"
@click="submit"
class="mr-4"
>
Register
</v-btn>
<v-btn color="secondary" @click="clear">
Clear
</v-btn>
</v-form>
</v-card-text>
</v-card>
</v-col>
</v-row>
</v-container>
</template>
<script>
export default {
data() {
return {
valid: false,
username: '',
email: '',
password: '',
confirmPassword: '',
usernameRules: [
(v) => !!v || 'Username is required',
(v) => v.length >= 3 || 'Username must be at least 3 characters',
],
emailRules: [
(v) => !!v || 'Email is required',
(v) => /.+@.+\..+/.test(v) || 'E-mail must be valid',
],
passwordRules: [
(v) => !!v || 'Password is required',
(v) => v.length >= 6 || 'Password must be at least 6 characters',
],
confirmPasswordRules: [
(v) => !!v || 'Password confirmation is required',
(v) => v === this.password || 'Passwords do not match',
],
};
},
methods: {
submit() {
if (this.$refs.form.validate()) {
// Implement registration logic here
alert('Account successfully registered!');
}
},
clear() {
this.$refs.form.reset();
this.password = '';
this.confirmPassword = '';
},
},
};
</script>
<style scoped>
.fill-height {
min-height: 100vh;
}
</style>
Explanation of the Code 코드 설명
- v-container, v-row, v-col: These Vuetify components are used to create a responsive layout, ensuring that the registration form is centered and adapts to different screen sizes.
v-container, v-row, v-col: 이러한 Vuetify 구성 요소는 반응형 레이아웃을 생성하는 데 사용되어 등록 양식이 중앙에 배치되고 다양한 화면 크기에 맞게 조정됩니다. - v-card: Wraps the form elements in a card layout, giving a clean and professional look.
v-card: 양식 요소를 카드 레이아웃으로 묶어 깔끔하고 전문적인 느낌을 줍니다. - v-card-title and v-card-text: Define sections of the card for the title and the content area where the form fields are placed.
v-card-title 및 v-card-text: 제목에 대한 카드 섹션과 양식 필드가 배치되는 콘텐츠 영역을 정의합니다. - v-form: Provides form validation capabilities. The lazy-validation prop allows validation to occur only when a field is blurred or on form submission.
v-form: 양식 유효성 검사 기능을 제공합니다. lazy-validation 소품을 사용하면 필드가 흐릿하거나 양식 제출 시에만 유효성 검사가 발생하도록 허용합니다. - v-text-field: Used to create input fields for the username, email, password, and password confirmation. Each field has appropriate validation rules.
v-text-field: 사용자 이름, 이메일, 비밀번호 및 비밀번호 확인을 위한 입력 필드를 만드는 데 사용됩니다. 각 필드에는 적절한 유효성 검사 규칙이 있습니다. - Validation Rules: 검증 규칙:
- usernameRules: Checks if the username is present and at least 3 characters long.
usernameRules: 사용자 이름이 존재하고 길이가 3자 이상인지 확인합니다. - emailRules: Ensures the email field is not empty and contains a valid email format.
emailRules: 이메일 필드가 비어 있지 않고 유효한 이메일 형식이 포함되어 있는지 확인합니다. - passwordRules: Checks that the password is not empty and has a minimum length.
passwordRules: 비밀번호가 비어 있지 않고 최소 길이인지 확인합니다. - confirmPasswordRules: Ensures the confirmation password matches the entered password.
confirmPasswordRules: 확인 비밀번호가 입력한 비밀번호와 일치하는지 확인합니다. - Data and Methods: 데이터 및 방법:
- valid: Tracks the form validation state.
valid: 양식 유효성 검사 상태를 추적합니다. - username, email, password, confirmPassword: Data bindings for the form inputs.
username, email, password, confirmPassword: 양식 입력에 대한 데이터 바인딩. - submit(): A method that validates the form and alerts the user upon successful validation. You can replace the alert with actual registration logic (e.g., API call).
submit(): 양식의 유효성을 검사하고 유효성 검사에 성공하면 사용자에게 경고하는 메서드입니다. 경고를 실제 등록 논리(예: API 호출)로 대체할 수 있습니다. - clear(): Resets the form inputs and validation state.
clear(): 양식 입력 및 유효성 검사 상태를 재설정합니다.
Step 3: Use the Register.vue Component
3단계: Register.vue 구성 요소 사용
To use this component in your main application, open src/App.vue and modify the template:
기본 애플리케이션에서 이 구성 요소를 사용하려면 src/App.vue를 열고 템플릿을 수정하세요.
<template>
<v-app>
<v-main>
<Register />
</v-main>
</v-app>
</template>
<script>
import Register from './components/Register.vue';
export default {
components: {
Register,
},
};
</script>
Step 4: Run Your Application
4단계: 애플리케이션 실행
To view the registration screen, run your Vue application:
등록 화면을 보려면 Vue 애플리케이션을 실행하세요.
npm run serve
Open your browser and navigate to http://localhost:8080 to see the registration form in action.
브라우저를 열고 'http://localhost:8080'으로 이동하여 실제 등록 양식을 확인하세요.
Additional Enhancements 추가 개선 사항
- Error Handling: Consider adding error messages to give users feedback if registration fails (e.g., due to existing email or server error).
오류 처리: 등록이 실패한 경우(예: 기존 이메일 또는 서버 오류로 인해) 사용자에게 피드백을 제공하기 위해 오류 메시지를 추가하는 것을 고려하세요. - Password Strength Indicator: Enhance the user experience by adding a password strength indicator.
비밀번호 강도 표시기: 비밀번호 강도 표시기를 추가하여 사용자 경험을 향상합니다. - Design Customization: Utilize Vuetify's design utilities to further customize the layout and design of the form to match your brand.
디자인 사용자 정의: Vuetify의 디자인 유틸리티를 활용하여 브랜드에 맞게 양식의 레이아웃과 디자인을 추가로 사용자 정의합니다. - Backend Integration: Connect the submit method with your backend API to handle user registration.
백엔드 통합: submit 메소드를 백엔드 API와 연결하여 사용자 등록을 처리합니다.
By following these steps, you’ve successfully created an account registration screen using Vuetify in a Vue.js project.
다음 단계를 따르면 Vue.js 프로젝트에서 Vuetify를 사용하여 계정 등록 화면을 성공적으로 만들었습니다.
ChatGPT가 시키는 대로 했습니다.
ChatGPT가 제시하는 코드를 실제 적용하면서 문제가 있는 부분들은 디버깅하여 코드를 수정하고 더 필요한 부분이 있으면 추가 합니다.
1. 실행
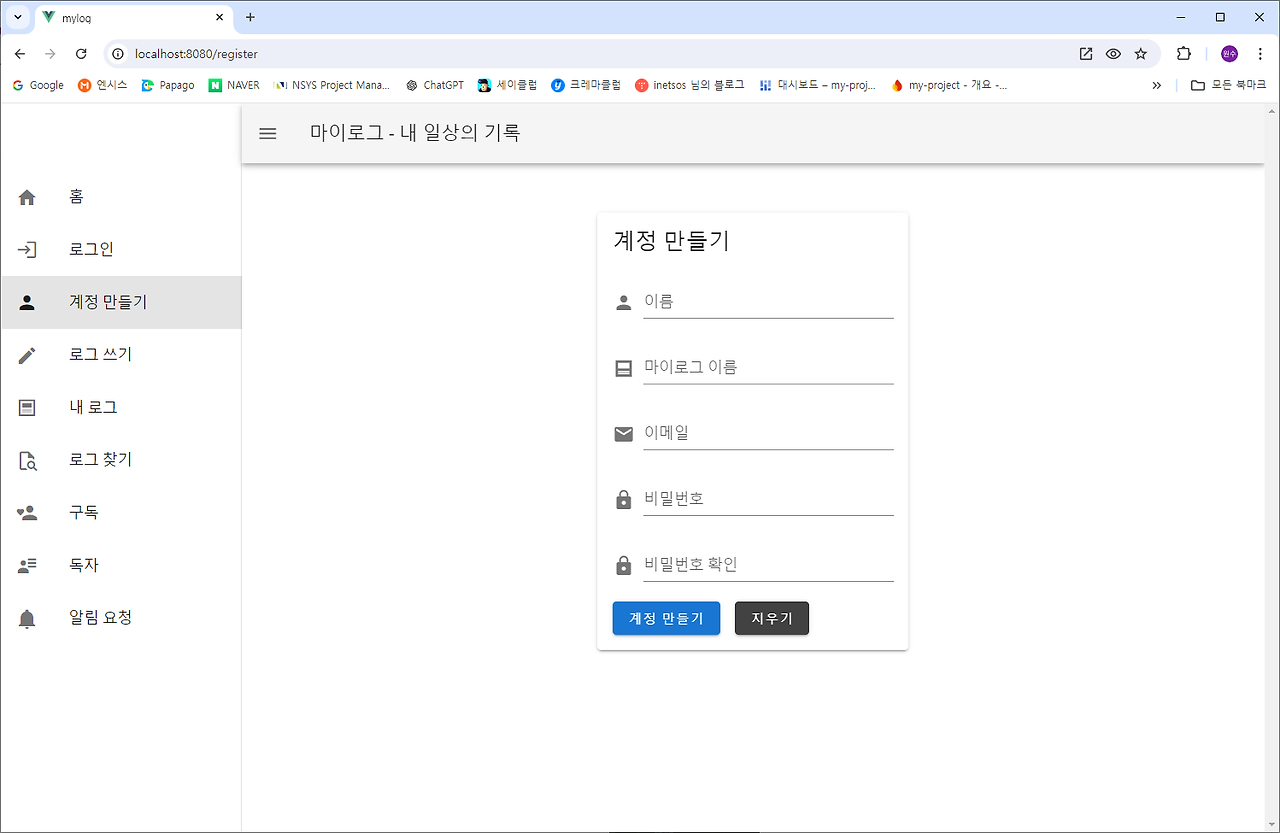
2. Source Code
src/views/RegisterView.vue
<!-- src/views/RegisterView.vue -->
<template>
<!-- v-container class="fill-height" fluid>
<v-row align="center" justify="center" -->
<v-container fluid>
<v-row align="center" justify="center" class="mt-4">
<v-col cols="12" sm="8" md="4">
<v-card>
<v-card-title class="text-h5">
계정 만들기
</v-card-title>
<v-card-text>
<v-form ref="form" v-model="valid" lazy-validation>
<v-text-field
v-model="username"
:rules="usernameRules"
label="이름"
prepend-icon="mdi-account"
required
></v-text-field>
<v-text-field
v-model="mylogname"
:rules="usernameRules"
label="마이로그 이름"
prepend-icon="mdi-post"
required
></v-text-field>
<v-text-field
v-model="email"
:rules="emailRules"
label="이메일"
prepend-icon="mdi-email"
required
></v-text-field>
<v-text-field
v-model="password"
:rules="passwordRules"
label="비밀번호"
prepend-icon="mdi-lock"
type="password"
required
></v-text-field>
<v-text-field
v-model="confirmPassword"
:rules="confirmPasswordRules"
label="비밀번호 확인"
prepend-icon="mdi-lock"
type="password"
required
></v-text-field>
<v-btn
:disabled="!valid"
color="primary"
@click="submit"
class="mr-4"
>
계정 만들기
</v-btn>
<v-btn color="secondary" @click="clear">
지우기
</v-btn>
</v-form>
</v-card-text>
</v-card>
</v-col>
</v-row>
</v-container>
</template>
<script>
export default {
data() {
return {
valid: false,
username: '',
mylogname: '',
email: '',
password: '',
confirmPassword: '',
usernameRules: [
(v) => !!v || 'Username is required',
(v) => (!!v && v.length >= 3) || 'Username must be at least 3 characters',
],
emailRules: [
(v) => !!v || 'Email is required',
(v) => (!!v && /.+@.+\..+/.test(v)) || 'E-mail must be valid',
],
passwordRules: [
(v) => !!v || 'Password is required',
(v) => (!!v && v.length >= 6) || 'Password must be at least 6 characters',
],
confirmPasswordRules: [
(v) => !!v || 'Password confirmation is required',
(v) => (!!v && v === this.password) || 'Passwords do not match',
],
};
},
methods: {
submit() {
if (this.$refs.form.validate()) {
// Implement registration logic here
alert('Account successfully registered!');
}
},
clear() {
this.$refs.form.reset();
this.password = '';
this.confirmPassword = '';
},
},
};
</script>
<style scoped>
.fill-height {
min-height: 100vh;
}
</style>
'Vue로 PWA 개발' 카테고리의 다른 글
6. mylog 계정 만들기 - firestore에 저장 (1) | 2024.10.07 |
---|---|
5. mylog 계정 만들기 - Firebase Auth. (1) | 2024.10.07 |
3. myLog 프로젝트 (0) | 2024.10.03 |
2. mylog layout (0) | 2024.10.03 |
1. myLog 개발 (1) | 2024.10.01 |