앞의 firestore crud 예제에 firebase auth기능을 추가하려고 합니다.
37. ChatGPT에게 물었습니다.
Add firebase auth to this example to show it
이 예제에 firebase auth를 추가하여 보여줘
ChatGPT
To add Firebase authentication to the previous Vue.js application example, we will modify the application to include user login and registration using Firebase Auth. We'll also restrict CRUD operations to authenticated users.
이전 Vue.js 애플리케이션 예제에 Firebase 인증을 추가하기 위해 Firebase 인증을 사용한 사용자 로그인 및 등록을 포함하도록 애플리케이션을 수정하겠습니다. 또한 CRUD 작업을 인증된 사용자로 제한합니다.
Project Structure
/src
/components
AddUser.vue
UserList.vue
Auth.vue
/store
index.js
firebase.js
App.vue
main.js
1. Initialize Firebase with Auth
Update the firebase.js file to include Firebase Auth:
Firebase 인증을 포함하도록 firebase.js 파일을 업데이트합니다.
src/firebase.js
// src/firebase.js
import firebase from 'firebase/compat/app';
import 'firebase/compat/auth';
import 'firebase/compat/firestore';
const firebaseConfig = {
apiKey: 'YOUR_API_KEY',
authDomain: 'YOUR_AUTH_DOMAIN',
projectId: 'YOUR_PROJECT_ID',
storageBucket: 'YOUR_STORAGE_BUCKET',
messagingSenderId: 'YOUR_MESSAGING_SENDER_ID',
appId: 'YOUR_APP_ID'
};
firebase.initializeApp(firebaseConfig);
const db = firebase.firestore();
const auth = firebase.auth();
export { db, auth };
2. Update Vuex Store
Update the store/index.js file to manage authentication state:
인증 상태를 관리하려면 store/index.js 파일을 업데이트하세요.
src/store/index.js
// src/store/index.js
import Vue from 'vue';
import Vuex from 'vuex';
import { db, auth } from '@/firebase';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
user: null,
users: [],
isLoading: false,
error: null
},
mutations: {
setUser(state, user) {
state.user = user;
},
setUsers(state, users) {
state.users = users;
state.isLoading = false;
},
setError(state, error) {
state.error = error;
state.isLoading = false;
},
setLoading(state, isLoading) {
state.isLoading = isLoading;
}
},
actions: {
async fetchUsers({ commit }) {
commit('setLoading', true);
try {
const usersSnapshot = await db.collection('users').get();
const users = usersSnapshot.docs.map(doc => ({ id: doc.id, ...doc.data() }));
commit('setUsers', users);
} catch (error) {
commit('setError', error.message);
}
},
async addUser({ dispatch }, user) {
try {
await db.collection('users').add(user);
dispatch('fetchUsers');
} catch (error) {
console.error('Error adding user:', error);
}
},
async deleteUser({ dispatch }, userId) {
try {
await db.collection('users').doc(userId).delete();
dispatch('fetchUsers');
} catch (error) {
console.error('Error deleting user:', error);
}
},
async updateUser({ dispatch }, { userId, user }) {
try {
await db.collection('users').doc(userId).update(user);
dispatch('fetchUsers');
} catch (error) {
console.error('Error updating user:', error);
}
},
async login({ commit }, { email, password }) {
try {
const { user } = await auth.signInWithEmailAndPassword(email, password);
commit('setUser', user);
} catch (error) {
commit('setError', error.message);
}
},
async register({ commit }, { email, password }) {
try {
const { user } = await auth.createUserWithEmailAndPassword(email, password);
commit('setUser', user);
} catch (error) {
commit('setError', error.message);
}
},
async logout({ commit }) {
await auth.signOut();
commit('setUser', null);
},
setUser({ commit }, user) {
commit('setUser', user);
}
},
getters: {
user: state => state.user,
users: state => state.users,
isLoading: state => state.isLoading,
error: state => state.error
}
});
3. Create Auth Component
Create a component for user login and registration:
사용자 로그인 및 등록을 위한 구성 요소를 만듭니다.
src/components/Auth.vue
<!-- src/components/Auth.vue -->
<template>
<v-container>
<v-form @submit.prevent="handleSubmit">
<v-text-field v-model="email" label="Email" required></v-text-field>
<v-text-field v-model="password" label="Password" type="password" required></v-text-field>
<v-btn type="submit" color="primary">{{ isLogin ? 'Login' : 'Register' }}</v-btn>
<v-btn @click="toggleMode" text>{{ isLogin ? 'Switch to Register' : 'Switch to Login' }}</v-btn>
</v-form>
<v-alert v-if="error" type="error">{{ error }}</v-alert>
</v-container>
</template>
<script>
import { mapGetters, mapActions } from 'vuex';
export default {
data() {
return {
email: '',
password: '',
isLogin: true
};
},
computed: {
...mapGetters(['error'])
},
methods: {
...mapActions(['login', 'register']),
handleSubmit() {
const action = this.isLogin ? 'login' : 'register';
this[action]({ email: this.email, password: this.password });
},
toggleMode() {
this.isLogin = !this.isLogin;
}
}
};
</script>
4. Update Main Application File
Update the App.vue file to include authentication:
인증을 포함하도록 App.vue 파일을 업데이트합니다.
src/App.vue
<!-- src/App.vue -->
<template>
<v-app>
<v-main>
<v-container>
<v-btn @click="logout" v-if="user">Logout</v-btn>
<v-container v-if="!user">
<auth></auth>
</v-container>
<v-container v-else>
<user-list></user-list>
<add-user></add-user>
</v-container>
</v-container>
</v-main>
</v-app>
</template>
<script>
import UserList from './components/UserList.vue';
import AddUser from './components/AddUser.vue';
import Auth from './components/Auth.vue';
import { mapGetters, mapActions } from 'vuex';
export default {
components: {
UserList,
AddUser,
Auth
},
computed: {
...mapGetters(['user'])
},
methods: {
...mapActions(['logout'])
},
created() {
this.$store.dispatch('fetchUsers');
this.$store.dispatch('setUser', this.$store.state.user);
}
};
</script>
5. Main Entry Point
Ensure you have Vuetify and Vuex set up in your main entry point:
기본 진입점에 Vuetify 및 Vuex가 설정되어 있는지 확인하세요.
// src/main.js
import Vue from 'vue';
import App from './App.vue';
import store from './store';
import vuetify from './plugins/vuetify'; // Ensure you have Vuetify setup
Vue.config.productionTip = false;
new Vue({
store,
vuetify,
render: h => h(App),
created() {
// Set up Firebase auth state change listener
const { dispatch } = this.$store;
auth.onAuthStateChanged(user => {
dispatch('setUser', user);
if (user) {
dispatch('fetchUsers');
}
});
}
}).$mount('#app');
6. Vuetify Setup
If you don't have Vuetify set up, you can install and configure it as follows:
Vuetify가 설정되어 있지 않은 경우 다음과 같이 설치하고 구성할 수 있습니다.
// src/plugins/vuetify.js
import Vue from 'vue';
import Vuetify from 'vuetify/lib';
Vue.use(Vuetify);
export default new Vuetify({});
And ensure you import the Vuetify styles in your main.js or App.vue:
그리고 main.js 또는 App.vue에서 Vuetify 스타일을 가져왔는지 확인하세요.
// src/main.js
import 'vuetify/dist/vuetify.min.css';
Explanation
설명
- Vuex Store: Manages the state for authentication and users, including login, registration, and CRUD operations.
Vuex Store: 로그인, 등록, CRUD 작업을 포함하여 인증 및 사용자 상태를 관리합니다. - Auth.vue: Contains forms for user login and registration using Firebase Auth.
Auth.vue: Firebase 인증을 사용한 사용자 로그인 및 등록을 위한 양식이 포함되어 있습니다 - AddUser.vue: Contains a form for adding new users to Firestore.
AddUser.vue: Firestore에 새 사용자를 추가하기 위한 양식이 포함되어 있습니다. - UserList.vue: Displays the list of users, shows appropriate messages when no data is available, and includes options to edit and delete users.
UserList.vue: 사용자 목록을 표시하고, 사용 가능한 데이터가 없을 때 적절한 메시지를 표시하며, 사용자 편집 및 삭제 옵션을 포함합니다. - App.vue: Main application file that integrates UserList, AddUser, and Auth components, with conditional rendering based on the authentication state.
App.vue: 'UserList', 'AddUser' 및 'Auth' 구성 요소를 인증 상태에 따른 조건부 렌더링과 통합하는 기본 애플리케이션 파일입니다. - Firebase Auth: Integrated to handle user login, registration, and maintaining authentication state.
Firebase 인증: 사용자 로그인, 등록, 인증 상태 유지를 처리하기 위해 통합되었습니다.
This example demonstrates a complete CRUD setup with Firestore, including user authentication, displaying a message when no data is available, and providing forms to add, edit, and delete users. Adjust the configuration and components according to your project structure and requirements.
이 예에서는 사용자 인증, 사용 가능한 데이터가 없을 때 메시지 표시, 사용자 추가, 편집, 삭제를 위한 양식 제공을 포함하여 Firestore를 사용한 완전한 CRUD 설정을 보여줍니다. 프로젝트 구조 및 요구 사항에 따라 구성 및 구성 요소를 조정합니다.
ChatGPT가 시키는 대로 했습니다.
1. 프로젝트 설정
vue create my-auth-1
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, PWA, Router, Vuex
? Choose a version of Vue.js that you want to start the project with 2.x
? Use history mode for router? (Requires proper server setup for index fallback in production) Yes
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated config files
? Save this as a preset for future projects? (y/N) n
2. vuetify 설치
cd my-auth-1
vue add vuetify
? Choose a preset: (Use arrow keys)
Vuetify 2 - Configure Vue CLI (advanced)
> Vuetify 2 - Vue CLI (recommended)
Vuetify 2 - Prototype (rapid development)
Vuetify 3 - Vite (preview)
Vuetify 3 - Vue CLI (preview)
3. Firebase 설정
npm install firebase

4. Initialize Firebase with Auth
src/firebase.js

5. Update Vuex Store
src/store/index.js

6. Create Auth Component
src/components/Auth.vue

7. Update Main Application File
src/App.vue

8. UserList.vue, AddUser.vue 복사하기
이전 예제와 연결된 예제라 이전 예제에서 사용된 컴포넌트를 가져옵니다.

9. 실행
npm run serve
로그인

등록
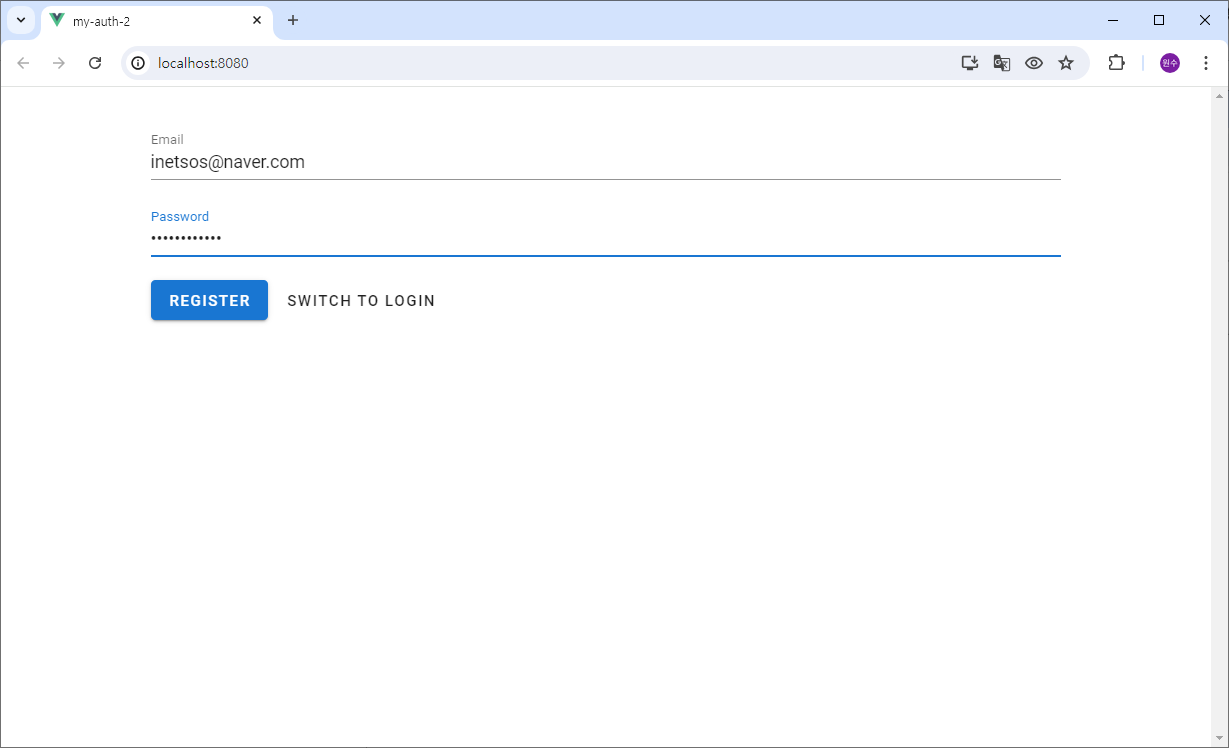
로그인 후
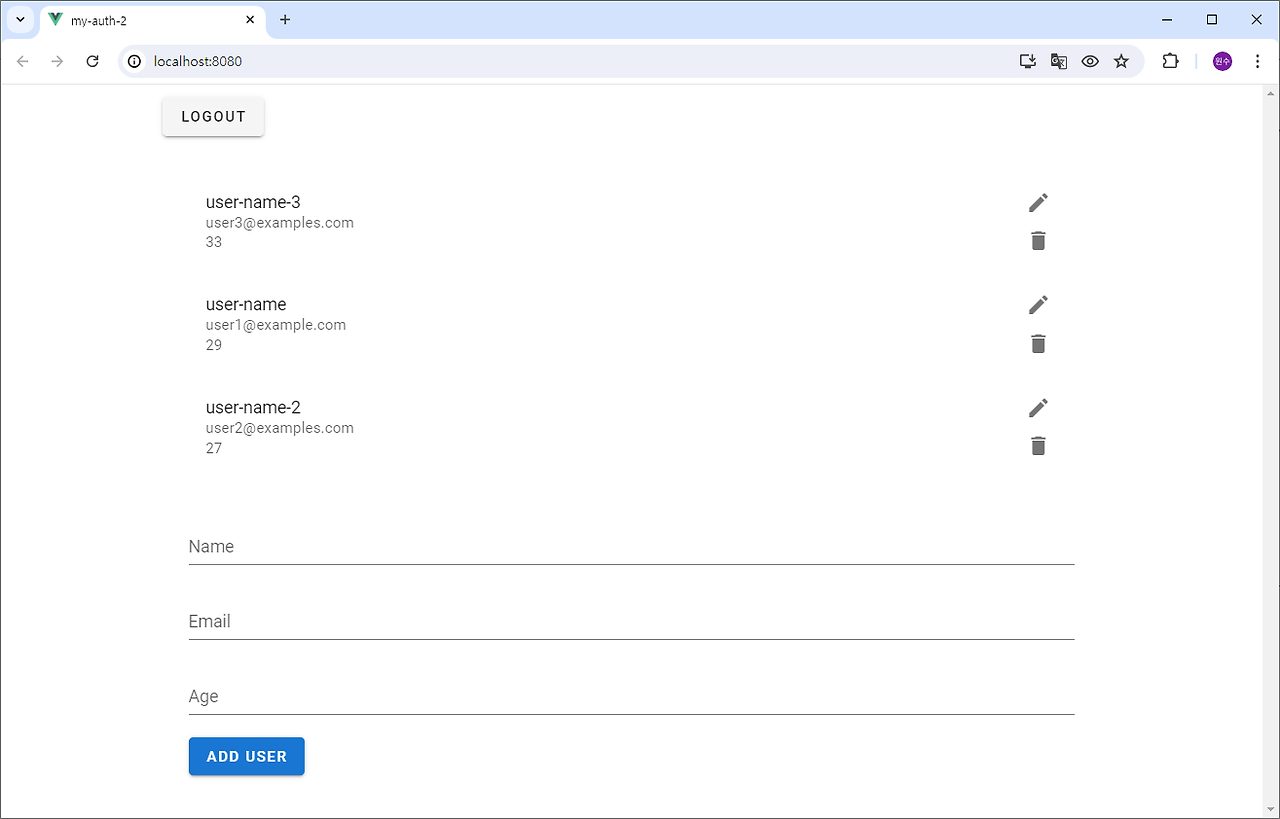
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. Firestore DB & Firebase Auth 4 (0) | 2024.07.31 |
---|---|
ChatGPT에게 물었습니다. Firestore DB & Firebase Auth 3 (0) | 2024.07.30 |
ChatGPT에게 물었습니다. Firestore DB & Firebase Auth 1 (0) | 2024.07.29 |
ChatGPT에게 물었습니다. Firestore CRUD 오류 수정 (0) | 2024.07.27 |
ChatGPT에게 물었습니다. Firestore CRUD 디버깅 (0) | 2024.07.27 |