22. ChatGPT가 시키는 대로 했습니다.
Vuex 설명 예제를 따라 했습니다.
1. 프로젝트 설정
vue create my-project-2
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, PWA, Router, Vuex
? Choose a version of Vue.js that you want to start the project with 2.x
? Use history mode for router? (Requires proper server setup for index fallback in production) Yes
? Where do you prefer placing config for Babel, ESLint, etc.? In dedicated config files
? Save this as a preset for future projects? (y/N) n
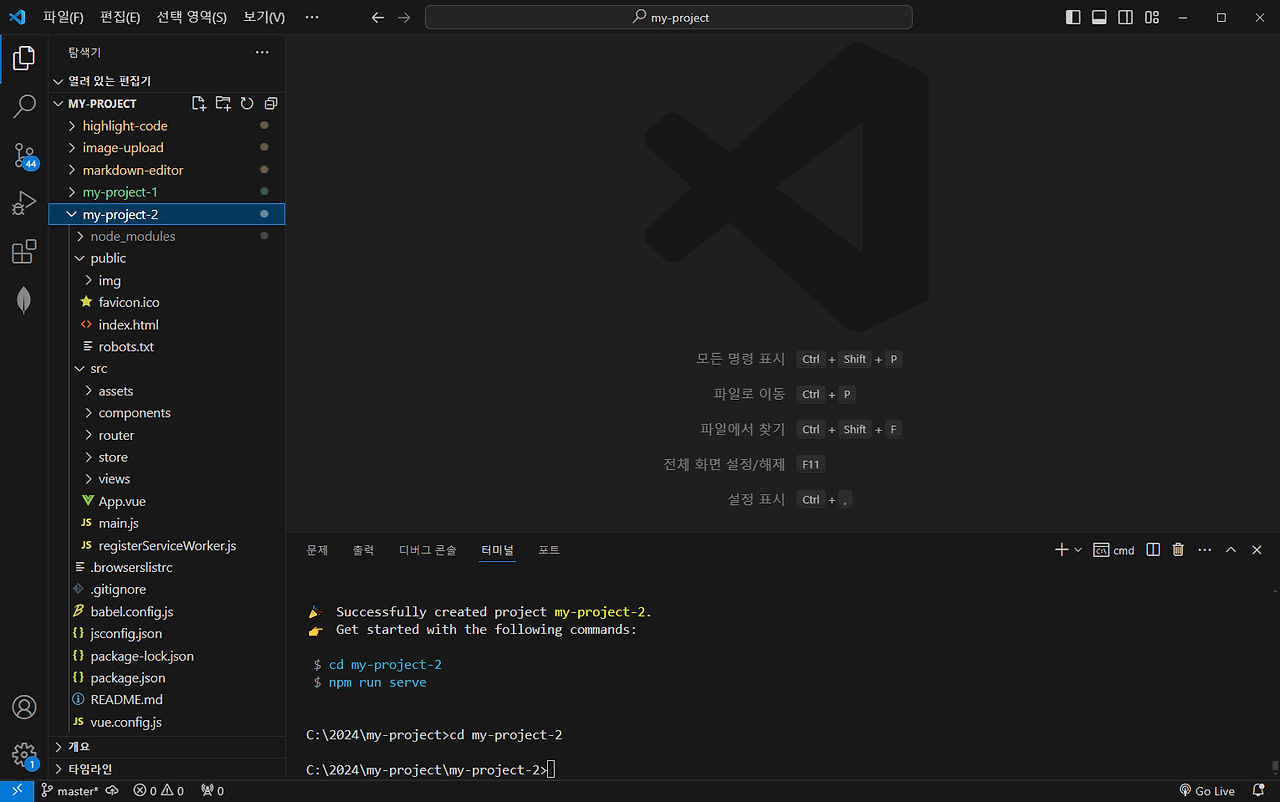
2. store/modules/counter.js
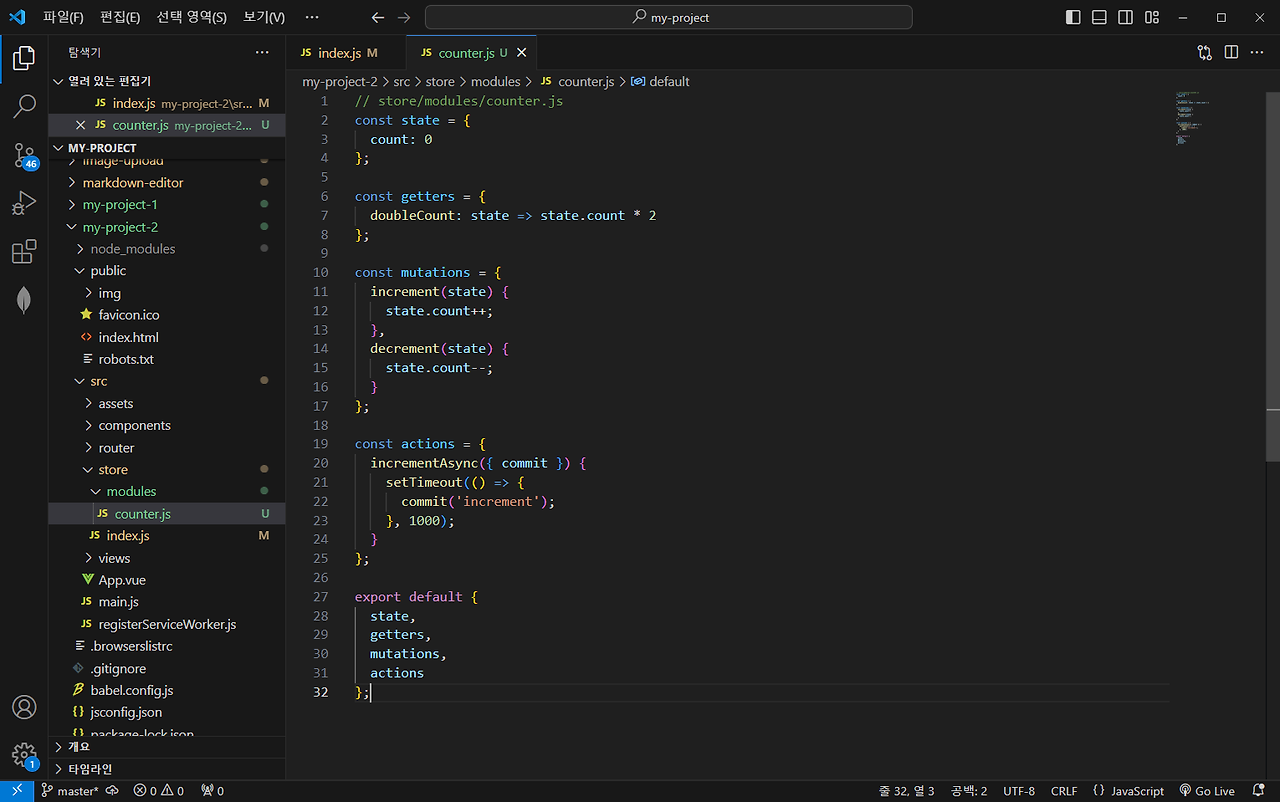
3. store/index.js
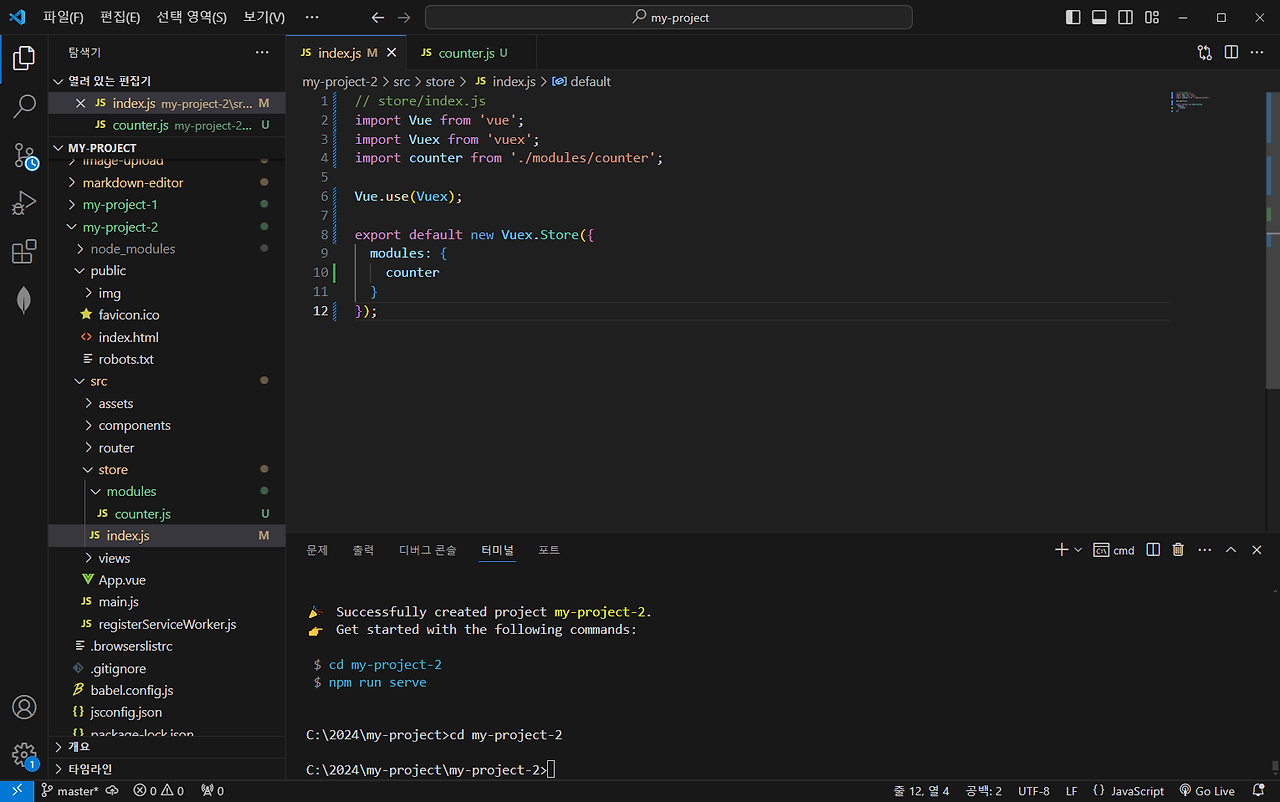
4. App.vue
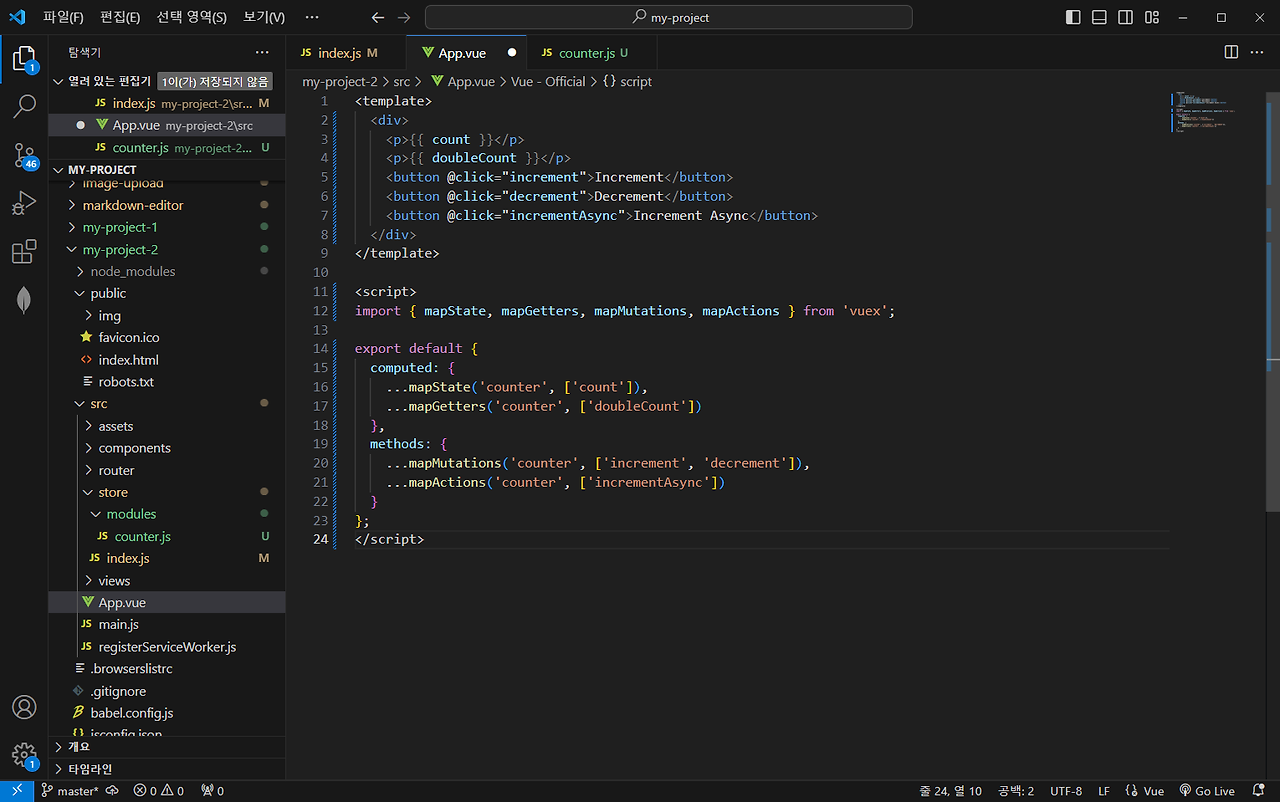
5. 실행
npm run serve
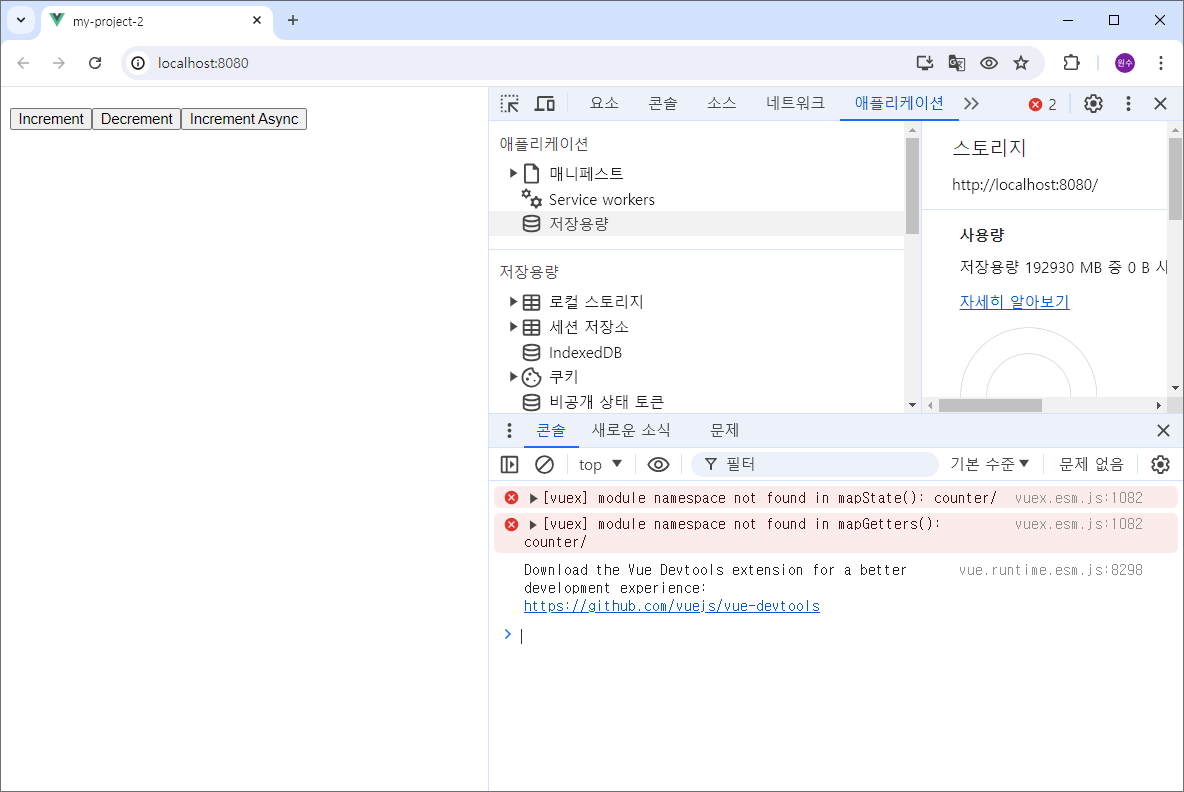
디버깅
[vuex] module namespace not found in mapState(): counter/
The error message module namespace not found in mapState(): counter/ usually indicates that the Vuex module is not correctly registered or the namespace is not correctly referenced. To resolve this issue, make sure the Vuex store module is properly defined, registered, and referenced.
‘[vuex] module namespace not found in mapState(): counter/’ 오류 메시지는 일반적으로 Vuex 모듈이 올바르게 등록되지 않았거나 네임스페이스가 올바르게 참조되지 않았음을 나타냅니다. 이 문제를 해결하려면 Vuex 저장소 모듈이 올바르게 정의, 등록 및 참조되었는지 확인하세요
Here’s a step-by-step guide to creating and using a namespaced Vuex module for a counter.
다음은 카운터용 네임스페이스 Vuex 모듈을 생성하고 사용하는 방법에 대한 단계별 가이드입니다.
Step 1: Create a Namespaced Module : 네임스페이스 모듈 생성
First, create a Vuex module for the counter with namespacing enabled.
먼저 네임스페이스가 활성화된 카운터에 대한 Vuex 모듈을 만듭니다.
// src/store/modules/counter.js
// src/store/modules/counter.js
const state = {
count: 0
};
const mutations = {
increment(state) {
state.count++;
},
decrement(state) {
state.count--;
}
};
const actions = {
incrementAsync({ commit }) {
setTimeout(() => {
commit('increment');
}, 1000);
}
};
const getters = {
doubleCount: state => state.count * 2
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
Step 2: Register the Module in the Vuex Store : Vuex 스토어에 모듈 등록
Next, register the module in your Vuex store.
다음으로 Vuex 스토어에 모듈을 등록하세요.
// src/store/index.js
// src/store/index.js
import Vue from 'vue';
import Vuex from 'vuex';
import counter from './modules/counter';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
counter
}
});
Step 3: Map State, Mutations, Actions, and Getters in Your Component : 구성 요소의 상태, 변형, 작업 및 게터 매핑
Now, use the mapState, mapMutations, mapActions, and mapGetters helpers in your component, and make sure to reference the counter namespace correctly.
이제 구성 요소에서 mapState, mapMutations, mapActions 및 mapGetters 도우미를 사용하고 counter 네임스페이스를 올바르게 참조하는지 확인하세요.
src/components/Counter.vue
<!-- src/components/Counter.vue -->
<template>
<v-container>
<v-row>
<v-col cols="12" sm="6" md="4">
<v-card>
<v-card-title>
<v-icon left>mdi-counter</v-icon>
Counter
</v-card-title>
<v-card-text>
<div>Count: {{ count }}</div>
<div>Double Count: {{ doubleCount }}</div>
</v-card-text>
<v-card-actions>
<v-btn color="primary" @click="increment">
<v-icon left>mdi-plus</v-icon>
Increment
</v-btn>
<v-btn color="secondary" @click="decrement">
<v-icon left>mdi-minus</v-icon>
Decrement
</v-btn>
<v-btn color="info" @click="incrementAsync">
<v-icon left>mdi-clock-outline</v-icon>
Increment Async
</v-btn>
</v-card-actions>
</v-card>
</v-col>
</v-row>
</v-container>
</template>
<script>
import { mapState, mapGetters, mapMutations, mapActions } from 'vuex';
export default {
name: 'Counter',
computed: {
...mapState('counter', ['count']),
...mapGetters('counter', ['doubleCount'])
},
methods: {
...mapMutations('counter', ['increment', 'decrement']),
...mapActions('counter', ['incrementAsync'])
}
};
</script>
<style>
/* Optional: Include additional styles */
</style>
Step 4: Use the Counter Component in Your App : 앱에서 카운터 구성요소 사용
Modify your App.vue to use the Counter component.
Counter 구성 요소를 사용하도록 App.vue를 수정하세요.
src/App.vue
<!-- src/App.vue -->
<template>
<v-app>
<v-main>
<counter />
</v-main>
</v-app>
</template>
<script>
import Counter from './components/Counter.vue';
export default {
name: 'App',
components: {
Counter
}
};
</script>
<style>
@import '~vuetify/dist/vuetify.min.css';
</style>
Step 5: Run Your Application : 애플리케이션 실행
Finally, run your application to test the counter functionality.
마지막으로 애플리케이션을 실행하여 카운터 기능을 테스트합니다.
npm run serve
Summary 요약
This guide demonstrates how to create and use a namespaced Vuex module in a Vue.js application. By ensuring that the module is correctly defined, registered, and referenced, you can avoid the module namespace not found error. The example shows a complete setup for a counter component using Vuetify for UI components and Vuex for state management.
이 가이드는 Vue.js 애플리케이션에서 네임스페이스된 Vuex 모듈을 생성하고 사용하는 방법을 보여줍니다. 모듈이 올바르게 정의, 등록 및 참조되었는지 확인하면 모듈 네임스페이스를 찾을 수 없음 오류를 피할 수 있습니다. 이 예에서는 UI 구성 요소에 Vuetify를 사용하고 상태 관리에 Vuex를 사용하는 카운터 구성 요소에 대한 전체 설정을 보여줍니다.
오류 수정
// src/store/modules/counter.js
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
src/store/modules/counter.js 파일의 export에 'namespaced: true,' 추가
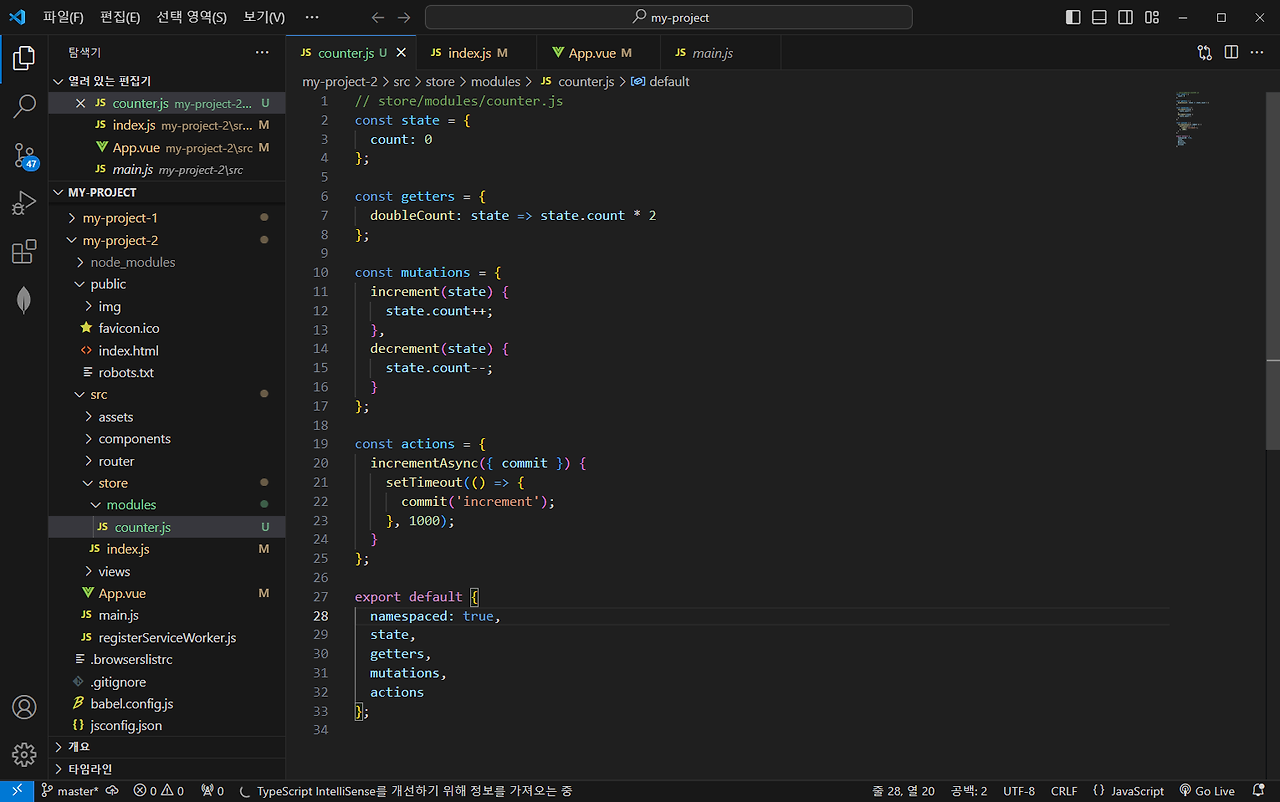
다시 실행
npm run serve
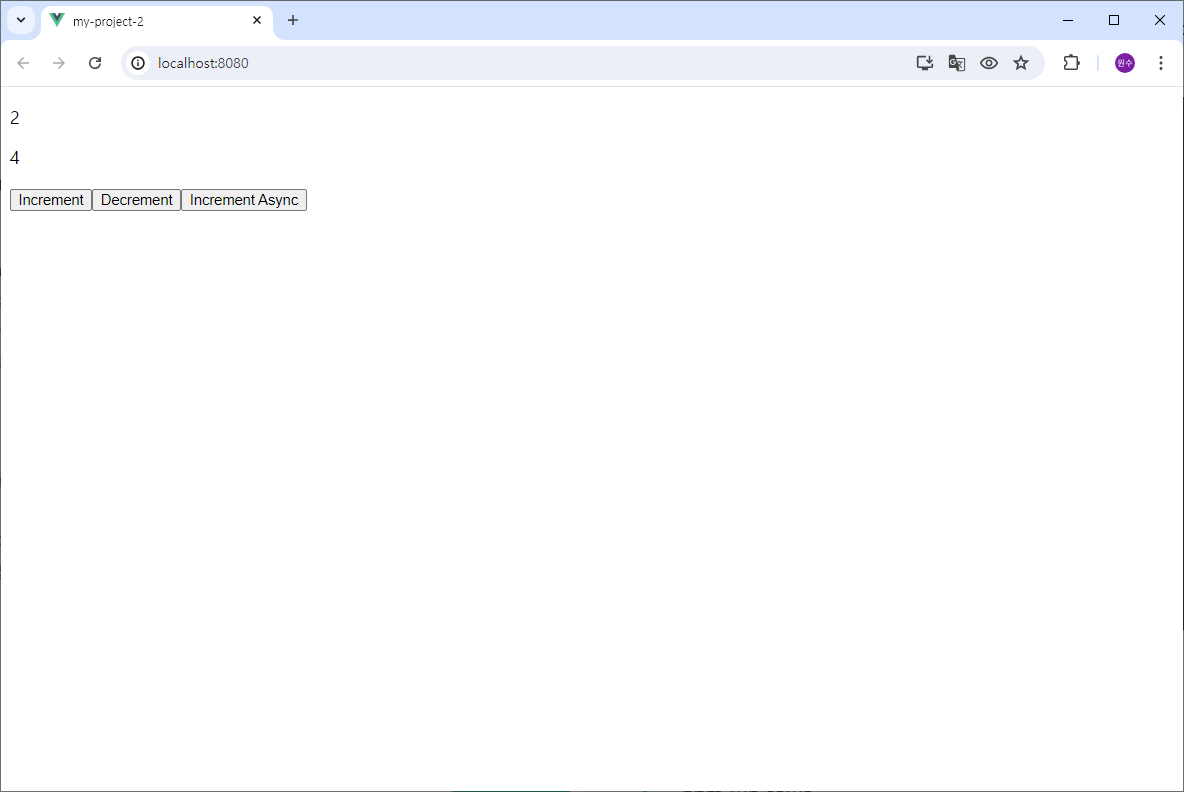
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. Vuetify (0) | 2024.07.21 |
---|---|
ChatGPT가 시키는 대로 했습니다. Vuex Example 2 (0) | 2024.07.21 |
ChatGPT에게 물었습니다. Vuex (0) | 2024.07.21 |
ChatGPT가 시키는 대로 했습니다. Vue Example (0) | 2024.07.13 |
ChatGPT에게 물었습니다. Vue (0) | 2024.07.13 |