마이로그 상세 보기 페이지에 구독 버튼이 있습니다. 마이로그의 저자에 대해 현재 구독 중이면 ‘구독 취소’가 현재 구독중이 아니면 ‘구독’ 버튼이 됩니다. 마이로그의 구독 버튼을 눌러 구독을 하게 되면 현재 보고 있는 마이로그 뿐 아니라 마이로그 저자에 대한 구독이므로 구독 저자의 모든 마이로그에 구독으로 표시됩니다. 즉 구독 중인 마이로그 저자의 각 마이로그 상세 보기에는 ‘구독 취소’버튼이 나타나는 것입니다. ‘구독 취소’는 구독 중이라는 뜻입니다. 구독 정보는 firestore의 subscriptions 컬렉션에 저장이 되고, 필드는 userId(회원 아이디), authorId(구독저자 아이디), createdat(구독 시작 날짜)가 있습니다. subscriptions 컬렉션에 문서가 있으면 구독 중이고 문서가 없으면 구독중이 아닙니다. 구독 취소를 하면 subscriptions에서 문서를 삭제합니다.
전체 회원, 전체 마이로그와 마찬가지로 전체 구독 정보는 상태에 로드 되어 있습니다. 지금 보고 있는 마이로그에 대해 구독중인 여부와 구독 id로 로드하고 있습니다. 그러므로 구독 정보 삭제할 때 구독 Id를 사용할 수 있습니다.
1. 마이로그 구독
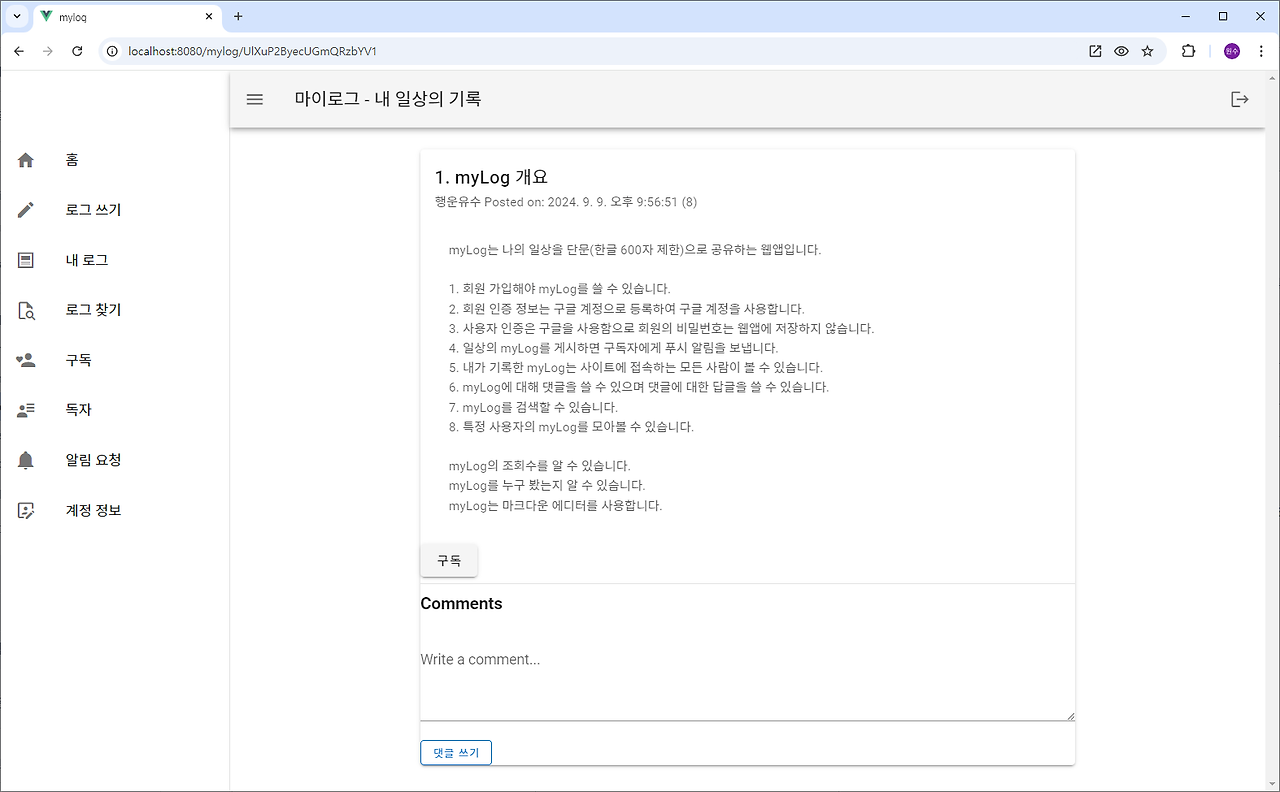
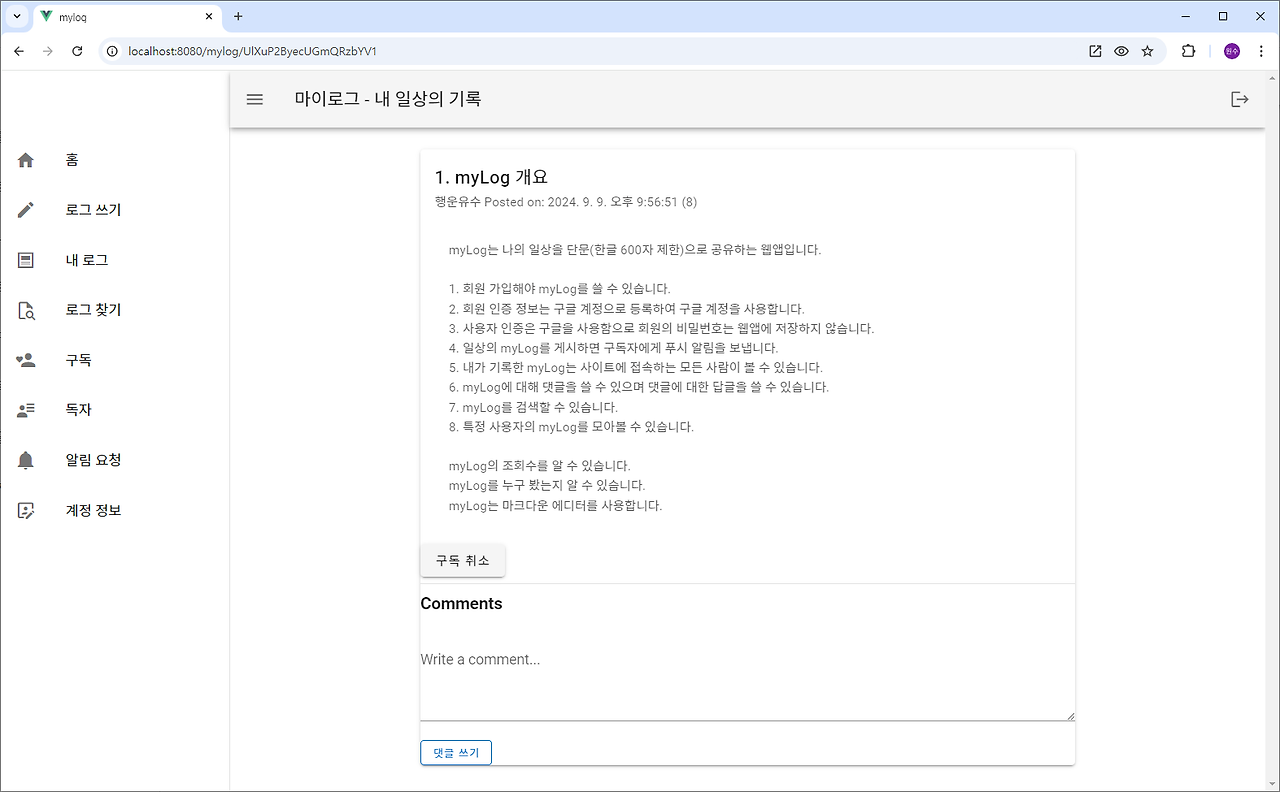
2. src/views/MyLogView.vue
<!-- src/views/MyLogView.vue -->
<template>
<v-container>
<v-row justify="center">
<v-col cols="12" md="8">
. . .
<v-btn @click="toggleSubscription">
{{ isSubscribed ? "구독 취소" : "구독" }}
</v-btn>
. . .
</v-row>
</v-container>
</template>
<script>
import { mapActions, mapGetters } from "vuex";
import sanitizeHtml from 'sanitize-html';
export default {
data() {
return {
content: '',
newComment: '',
newReply: '',
replyingTo: null
};
},
async created() {
. . .
const authorId = this.mylog.userId;
if(userId) {
this.checkSubscription({userId, authorId}); // Check if the user is subscribed when the component mounts
}
},
computed: {
...mapGetters('mylogs',['mylog', 'comments', 'isSubscribed', 'subscriptionId']),
. . .
},
methods: {
...mapActions('mylogs', ['fetchMylog', 'resetError', 'updateViewCount','addComment', 'fetchComments','deleteComment',
'addReply', 'deleteReply', 'checkSubscription', 'subscribeToUser', 'unsubscribeFromUser']),
. . .
// -- 구독 -------------------------------
// Toggle subscription: subscribe if not subscribed, unsubscribe if already subscribed
async toggleSubscription() {
const userId = this.$store.state.auth.user.id;
const authorId = this.mylog.userId;
const subscriptionId = this.subscriptionId;
if (this.isSubscribed) {
// Unsubscribe the user
await this.unsubscribeFromUser({userId, subscriptionId});
} else {
// Subscribe the user
await this.subscribeToUser({userId, authorId});
}
// 변경 내용 갱신을 위하여
this.checkSubscription({userId, authorId}); // Check if the user is subscribed when the component mounts
},
// -- 구독 끝 ----------------------------
}
};
</script>
3. src/store/modules/mylogs.js
// src/store/modules/mylogs.js
import router from '@/router'; // Vue Router import
import { v4 as uuidv4 } from 'uuid';
import { db, doc, collection, getDoc, getDocs, addDoc, setDoc, deleteDoc, query, orderBy, updateDoc, arrayUnion, increment, where } from "@/firebase";
const state = {
. . .
subscriptions: [], // 구독
isSubscribed: false, // 구독 여부 - 마이로그 상세 보기에서 사용
subscriptionId: '', // 구독 등록 Id - 구독정보 삭제에 사용
. . .
};
const mutations = {
. . .
setSubscriptions(state, subscriptions) { // 구독
state.subscriptions = subscriptions;
},
setSubscribed(state, subscribed) { // 구독 여부
state.isSubscribed = subscribed;
},
setSubscriptionId(state, subscriptionId) { // 구독 Id
state.subscriptionId = subscriptionId;
},
};
const actions = {
. . .
// -- 구독 ----------------------------------
// 구독 여부 확인
async checkSubscription({ commit }, { userId, authorId }) {
const q = query(
collection(db, "subscriptions"),
where("userId", "==", userId),
where("authorId", "==", authorId)
);
const querySnapshot = await getDocs(q);
if (!querySnapshot.empty) {
commit("setSubscribed", true);
commit("setSubscriptionId", querySnapshot.docs[0].id); // Get the Firestore document ID for the subscription
} else {
commit("setSubscribed", false);
commit("setSubscriptionId", '');
}
},
// user에 대한 구독 로드
async fetchUserSubscriptions({ commit }, userId) {
const subscriptionRef = collection(db, "subscriptions");
const q = query(subscriptionRef, where("userId", "==", userId));
const querySnapshot = await getDocs(q);
const subscriptions = [];
querySnapshot.forEach((doc) => {
subscriptions.push(doc.data());
});
commit("setSubscriptions", subscriptions);
},
// 구독
async subscribeToUser({ commit, dispatch }, {userId, authorId}) {
//console.log('userId: ',userId )
//console.log('authorId: ',authorId )
try {
const subscriptionRef = collection(db, "subscriptions");
await addDoc(subscriptionRef, {
userId: userId,
authorId: authorId,
createdAt: new Date(),
});
dispatch("fetchUserSubscriptions", userId);
} catch (error) {
commit("setError", error);
console.error("Error subscribing:", error);
}
},
// 구독 해지
async unsubscribeFromUser({ commit, dispatch }, {userId, subscriptionId}) {
console.log('subscriptionId: ',subscriptionId )
try {
const subscriptionDocRef = doc(db, "subscriptions", subscriptionId);
await deleteDoc(subscriptionDocRef);
dispatch("fetchUserSubscriptions", userId);
} catch (error) {
commit("setError", error);
console.error("Error unsubscribing:", error);
}
},
// -- 구독 끝 -------------------------------
};
const getters = {
. . .
isSubscribed: state => state.isSubscribed, // 구독 여부 - 마이로그 상세 보기에서 사용
subscriptionId: state => state.subscriptionId, // 구독 등록 Id - 구독정보 삭제에 사용
. . .
};
export default {
namespaced: true,
state,
mutations,
actions,
getters
};
'Vue로 PWA 개발' 카테고리의 다른 글
41. mylog 회원별 보기 (0) | 2024.10.29 |
---|---|
40. mylog 구독 보기 (0) | 2024.10.28 |
38. mylog 구독 취소 (3) | 2024.10.28 |
37. mylog 구독 (0) | 2024.10.28 |
36. mylog 찾기 구현 (0) | 2024.10.28 |