14. ChatGPT에게 물었습니다.
Vuetify를 사용하여 OAuth 로그인 화면을 개선하고 싶습니다.
Vuetify와 material icon을 이용하여 화면을 꾸미고 싶어.
I want to decorate the screen using vuetify and material icon.
To decorate your Vue.js application using Vuetify and Material Icons, you'll need to follow these steps:
Vuetify 및 Material Icons를 사용하여 Vue.js 애플리케이션을 장식하려면 다음 단계를 따라야 합니다.
Step 1: Install Vuetify Vuetify 설치
If you don't already have Vuetify installed in your Vue project, you can add it using the Vue CLI:
Vue 프로젝트에 Vuetify가 아직 설치되어 있지 않은 경우 Vue CLI를 사용하여 추가할 수 있습니다.
vue add vuetify
During the installation, you can choose the default preset or configure it according to your preferences.
설치하는 동안 기본 사전 설정을 선택하거나 기본 설정에 따라 구성할 수 있습니다.
Step 2: Configure Vuetify Vuetify 구성
Ensure Vuetify is properly configured in your main.js or main.ts file. This file is usually located in the src directory of your Vue project.
main.js 또는 main.ts 파일에 Vuetify가 올바르게 구성되어 있는지 확인하세요. 이 파일은 일반적으로 Vue 프로젝트의 src 디렉토리에 있습니다.
// src/main.js
import { createApp } from 'vue';
import App from './App.vue';
import vuetify from './plugins/vuetify'; // Vuetify plugin configuration
import '@mdi/font/css/materialdesignicons.css'; // Import Material Design Icons
createApp(App)
.use(vuetify)
.mount('#app');
Next, create a vuetify.js file in the src/plugins directory (create the directory if it doesn't exist):
다음으로 src/plugins 디렉터리에 vuetify.js 파일을 만듭니다(없는 경우 디렉터리를 만듭니다).
// src/plugins/vuetify.js
import { createVuetify } from 'vuetify';
import 'vuetify/styles';
export default createVuetify({
// Add any global Vuetify configuration here
});
Step 3: Create a User Authentication Component with Vuetify Vuetify를 사용하여 사용자 인증 구성 요소 만들기
Now, create a Vuetify-based component to handle user authentication and display user information. Let's modify the previous UserAuth.vue component to use Vuetify and Material Icons:
이제 사용자 인증을 처리하고 사용자 정보를 표시하는 Vuetify 기반 구성 요소를 만듭니다. Vuetify 및 머티리얼 아이콘을 사용하도록 이전 UserAuth.vue 구성 요소를 수정해 보겠습니다.
<template>
<v-app>
<v-container>
<v-row justify="center">
<v-col cols="12" md="6">
<v-card class="pa-5">
<v-card-title>
<span class="headline">User Authentication</span>
</v-card-title>
<v-card-actions>
<v-btn color="primary" @click="login">
<v-icon left>mdi-google</v-icon>
Login with Google
</v-btn>
</v-card-actions>
<v-divider></v-divider>
<div v-if="user" class="mt-3">
<v-card-title class="headline">User Info</v-card-title>
<v-list-item>
<v-list-item-content>
<v-list-item-title><strong>Name:</strong> {{ user.displayName }}</v-list-item-title>
<v-list-item-subtitle><strong>Email:</strong> {{ user.email }}</v-list-item-subtitle>
</v-list-item-content>
<v-list-item-avatar>
<v-img :src="user.photoURL" alt="User Photo"></v-img>
</v-list-item-avatar>
</v-list-item>
</div>
</v-card>
</v-col>
</v-row>
</v-container>
</v-app>
</template>
<script>
import { ref } from 'vue';
import { auth, provider, signInWithPopup } from '../firebaseConfig';
export default {
name: 'UserAuth',
setup() {
const user = ref(null);
const login = async () => {
try {
const result = await signInWithPopup(auth, provider);
user.value = result.user;
} catch (error) {
console.error('Error during sign-in:', error);
}
};
return {
user,
login,
};
},
};
</script>
<style>
/* Add any custom styles here */
</style>
Step 4: Use the Component in Your App 앱에서 구성요소 사용
Update your App.vue to use the UserAuth component:
UserAuth 구성 요소를 사용하려면 App.vue를 업데이트하세요.
<template>
<v-app>
<v-main>
<UserAuth />
</v-main>
</v-app>
</template>
<script>
import UserAuth from './components/UserAuth.vue';
export default {
name: 'App',
components: {
UserAuth,
},
};
</script>
<style>
/* Add any custom styles here */
</style>
Explanation 설명
1. Vuetify Components: Vuetify 구성요소:
- Used Vuetify components like v-app, v-container, v-row, v-col, v-card, v-btn, v-icon, v-divider, and v-list-item to structure the layout and style the component.
v-app, v-container, v-row, v-col, v-card, v-btn, v-icon, v-와 같은 Vuetify 구성 요소를 사용했습니다. Divider 및 v-list-item을 사용하여 레이아웃을 구조화하고 구성 요소의 스타일을 지정합니다. - Added Material Design Icons by including the mdi-google icon for the Google login button.
Google 로그인 버튼에 'mdi-google' 아이콘을 포함시켜 머티리얼 디자인 아이콘을 추가했습니다.
2. Styling: 스타일링:
- Added padding and spacing using Vuetify's utility classes.
Vuetify의 유틸리티 클래스를 사용하여 패딩 및 간격을 추가했습니다. - Used Vuetify's responsive grid system to center the card and ensure it looks good on various screen sizes.
Vuetify의 반응형 그리드 시스템을 사용하여 카드를 중앙에 배치하고 다양한 화면 크기에서 잘 보이는지 확인했습니다.
3. Interactivity: 상호작용:
- The login method triggers the sign-in process and updates the user information in the component's state.
login 메소드는 로그인 프로세스를 트리거하고 구성요소 상태의 사용자 정보를 업데이트합니다. - The user information is conditionally displayed using Vue's v-if directive.
사용자 정보는 Vue의 'v-if' 지시문을 사용하여 조건부로 표시됩니다.
By following these steps, you can create a visually appealing user authentication component using Vuetify and Material Icons in your Vue.js application. This approach leverages Vuetify's powerful UI components and styling capabilities to enhance the user experience.
다음 단계를 따르면 Vue.js 애플리케이션에서 Vuetify 및 Material Icons를 사용하여 시각적으로 매력적인 사용자 인증 구성 요소를 만들 수 있습니다. 이 접근 방식은 Vuetify의 강력한 UI 구성 요소와 스타일링 기능을 활용하여 사용자 경험을 향상시킵니다.
OAuth 로그인 개선
Vuetify는 프로젝트를 만들고 각종 프로그램을 설치할 때 이미 설치를 하였습니다.
vue add vuetify
1. main.js
// src/main.js
import Vue from 'vue'
import App from './App.vue'
import './registerServiceWorker'
import router from './router'
import store from './store'
import vuetify from './plugins/vuetify'
Vue.config.productionTip = false
new Vue({
router,
store,
vuetify,
render: h => h(App)
}).$mount('#app')
2. vuetify.js
// src/plugins/vuetify.js
import Vue from 'vue';
import Vuetify from 'vuetify/lib/framework';
Vue.use(Vuetify);
export default new Vuetify({
});
3. UserAuth.vue
위의 코드 사용
4. App.vue
위의 코드 사용
5. 개선 후
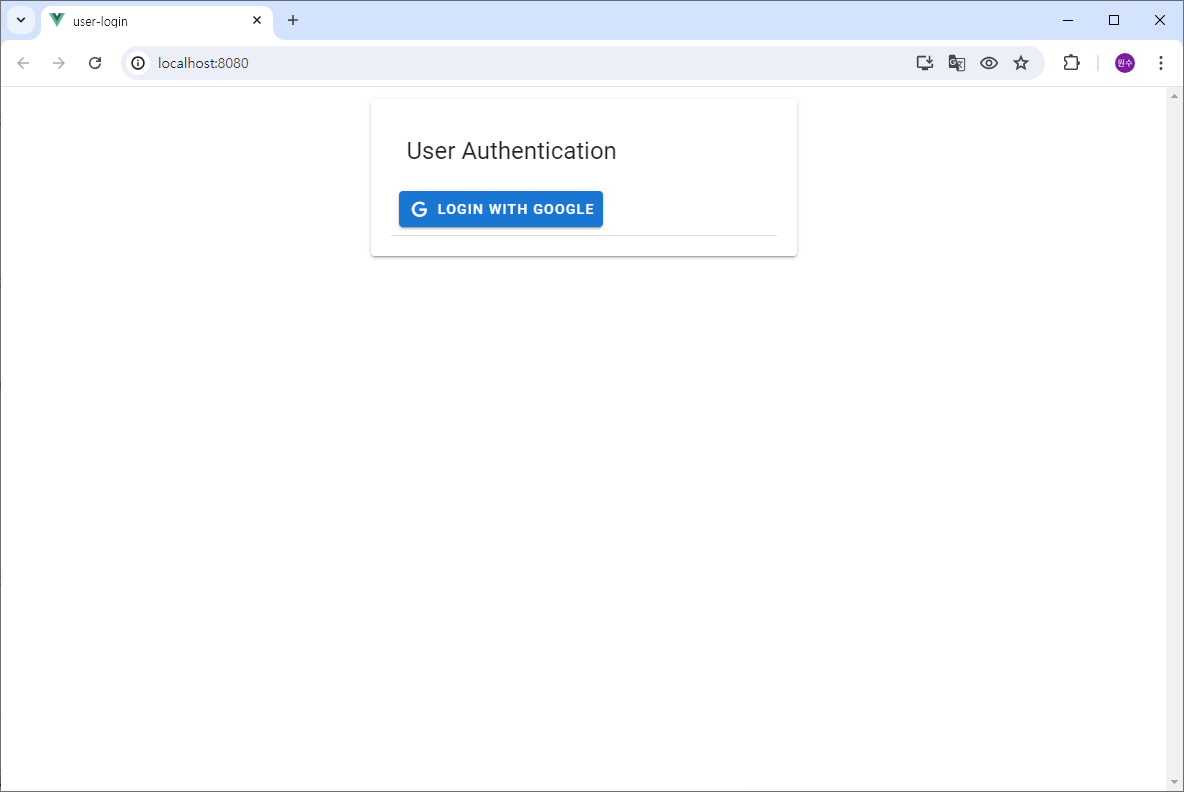
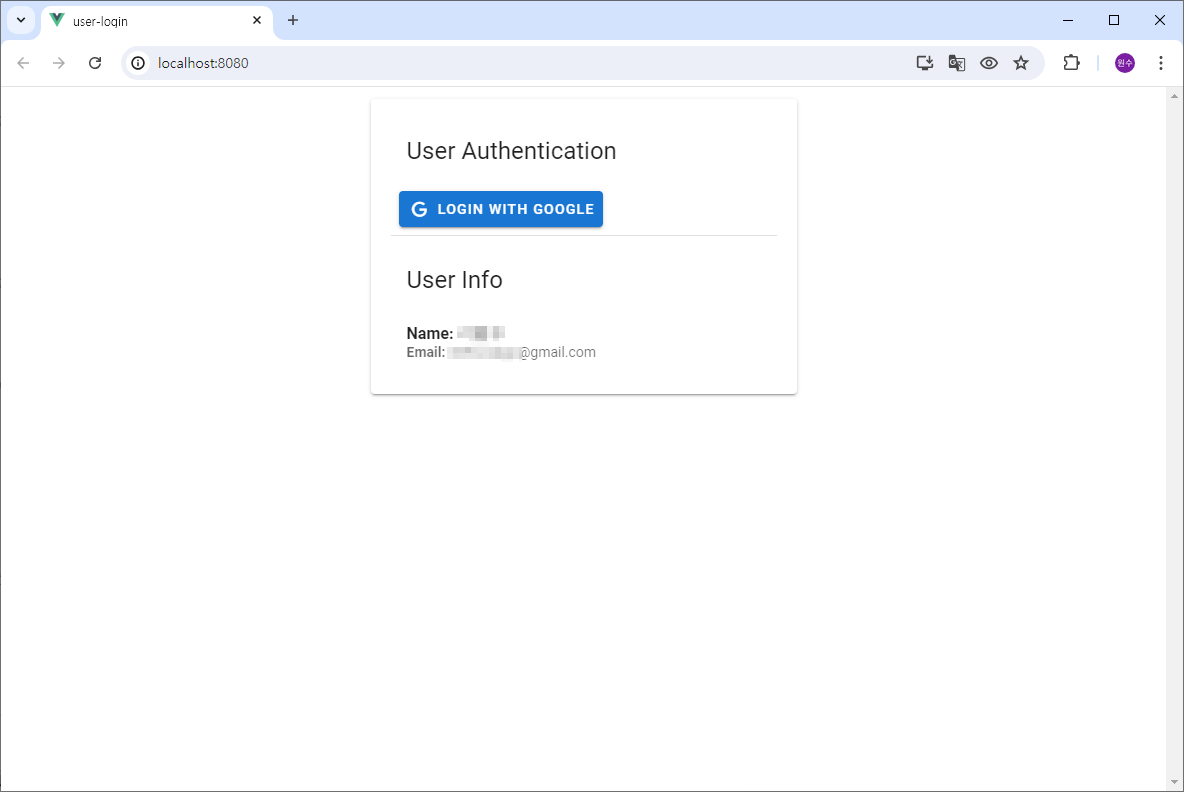
'PWA' 카테고리의 다른 글
ChatGPT에게 물었습니다. PWA의 기술 (0) | 2024.07.13 |
---|---|
ChatGPT에게 물었습니다. Firestore (0) | 2024.07.13 |
ChatGPT가 시키는 대로 했습니다. OAuth 로그인 (0) | 2024.07.13 |
ChatGPT에게 물었습니다. OAuth 인증 (0) | 2024.07.13 |
ChatGPT에게 물었습니다. PWA (0) | 2024.07.13 |