사용자가 웹앱에 접속하면 마이로그는 홈페이지를 보여줍니다. 마이로그가 사용자에게 홈페이지를 보여주기까지 등록된 전체 마이로그를 로드하고, 등록된 전체 회원 정보도 로드하고, 자동 로그인을 통해 로그인 한 사용자의 계정 정보로 로드합니다. 물론 이전에 로그아웃을 하였거나, 이전에 로그인한 경우가 없는 사용자라면 현재 접속한 사용자 정보는 없습니다. 웹앱이 홈페이지를 사용자에 보여주기까지의 과정을 살펴봅시다.
src/main.js
// src/main.js
import Vue from 'vue'
import App from './App.vue'
import './registerServiceWorker'
import router from './router'
import store from './store'
import vuetify from './plugins/vuetify'
Vue.config.productionTip = false
new Vue({
router,
store,
vuetify,
render: h => h(App),
created() {
// Set up Firebase auth state change listener
const { dispatch } = this.$store;
// Initialize Firebase authentication to check for the logged-in user
dispatch('auth/initializeAuth'); // 1
dispatch('auth/fetchUsers'); // 2
dispatch('mylogs/fetchMylogs'); // 3
}
}).$mount('#app')
1. dispatch('auth/initializeAuth');
- onAuthStateChanged : Firebase Authentication에서 로그인 상태 변화를 실시간으로 감지하는 메서드입니다.
- fetchUserWithUid : onAuthStateChanged로 자동 로그인되면 구글 계정의 user 객체를 받고, user.uid로 마이로그 웹앱의 계정 정보를 가져와 로그인 설정을 합니다.
initializeAuth({ commit, dispatch }) {
onAuthStateChanged(auth, (user) => {
if (user) {
// user.uid로 웹앱의 firestore DB에서 계정 정보를 가져온다.
dispatch('fetchUserWithUid', {uid: user.uid});
} else {
commit("setUser", null);
commit("setUserInfo", null);
}
});
},
async fetchUserWithUid({ commit, dispatch }, {uid}) {
// 로그인은 google 계정으로 한다.
// user 정보는 mylog 계정 정보를 사용한다.
// 그러므로 구글 계정의 uid로 mylog 계정의 user 정보를 가져와야 한다.
try {
const users = [];
const userRef = query(collection(db, "users"), where('uids', 'array-contains', uid));
const querySnapshot = await getDocs(userRef);
querySnapshot.forEach((doc) => {
// doc.data() is never undefined for query doc snapshots
users.push({ id: doc.id, ...doc.data() });
});
// 로그인 설정을 한다.
commit('setUser', users[0]);
dispatch("fetchUserInfo", users[0]);
} catch (error) {
console.error('Error fetching user:', error);
}
},
2. dispatch('auth/fetchUsers');
firestore에서 등록된 모든 계정 정보를 메모리에 로드합니다.
async fetchUsers({ commit }) {
commit('setLoading', true);
try {
const users = [];
const userRef = collection(db, "users");
const querySnapshot = await getDocs(userRef);
querySnapshot.forEach((doc) => {
// doc.data() is never undefined for query doc snapshots
users.push({ id: doc.id, ...doc.data() });
});
commit('setUsers', users);
} catch (error) {
commit('setError', error.message);
}
},
3. dispatch('mylogs/fetchMylogs')
firestore에 저장된 모든 마이로그를 메모리에 로드합니다.
async fetchMylogs({ commit }) {
commit('setLoading', true);
try {
const mylogs = [];
const mylogsRef = query(collection(db, "mylogs"), orderBy("createdAt", "desc"));
const querySnapshot = await getDocs(mylogsRef);
querySnapshot.forEach((doc) => {
// doc.data() is never undefined for query doc snapshots
mylogs.push({ id: doc.id, ...doc.data() });
});
commit('setMylogs', mylogs);
} catch (error) {
commit('setError', error);
} finally {
commit('setLoading', false);
}
},
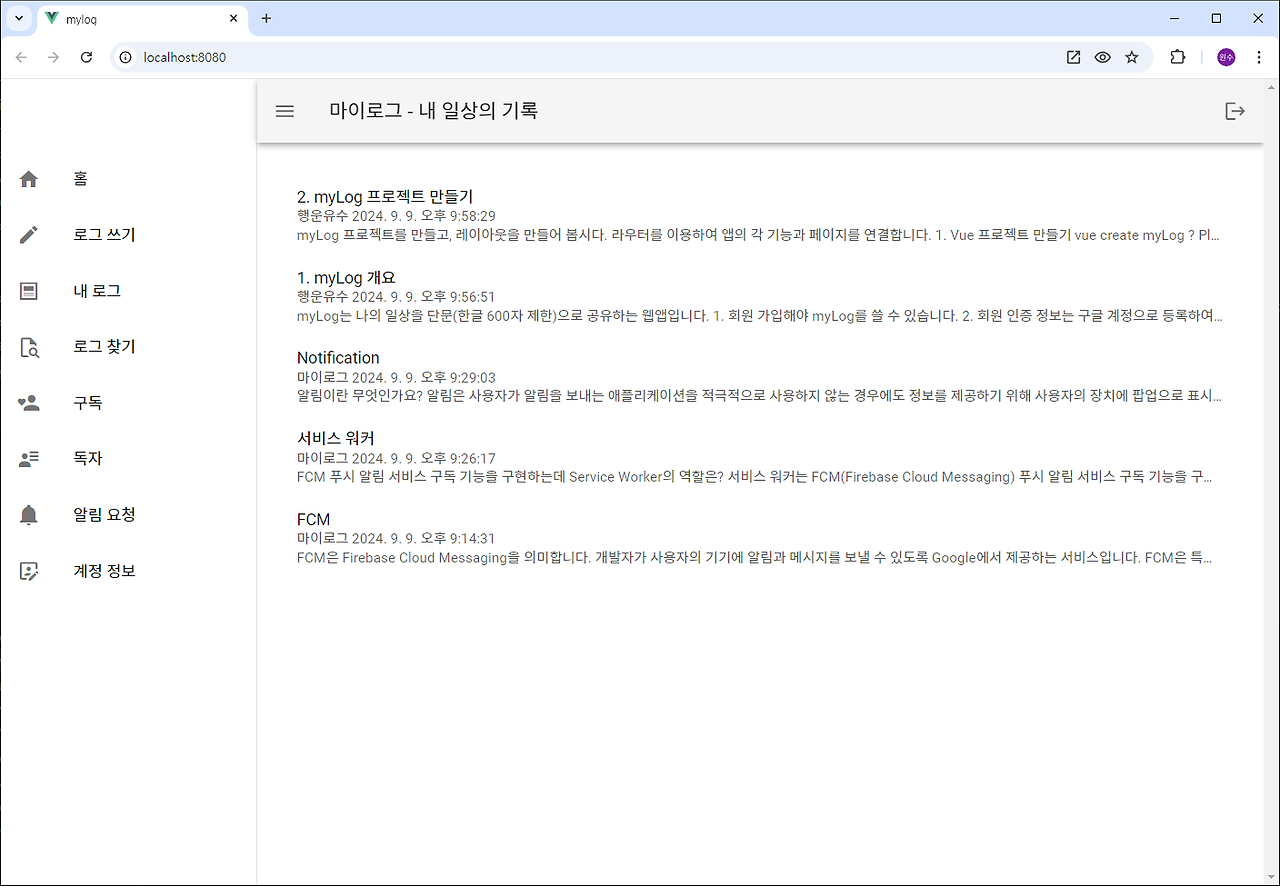
'Vue로 PWA 개발' 카테고리의 다른 글
22. mylog 쓰기 (0) | 2024.10.23 |
---|---|
21. mylog 홈페이지 (0) | 2024.10.22 |
19. mylog 자동 로그인 (0) | 2024.10.22 |
18. mylog 줄바꿈 (0) | 2024.10.19 |
17. mylog 보기 (1) | 2024.10.19 |