52. 글쓰기
ChatGPT에게 물었습니다.
Please explain in detail so that I can implement the function of writing a blog post and viewing the list of saved posts.
블로그에 글을 쓰고 저장된 글들의 리스트를 볼 수 있는 기능을 구현할 수 있도록 상세하게 설명 해줘.
Certainly! Let's walk through the steps to implement the functionality of writing a blog post and viewing the list of saved posts using Vue.js, Vuex, Vuetify, and Firestore.
넵! Vue.js, Vuex, Vuetify 및 Firestore를 사용하여 블로그 게시물을 작성하고 저장된 게시물 목록을 보는 기능을 구현하는 단계를 살펴보겠습니다.
Step-by-Step Guide
- Setup Vue and Vuetify
Vue 및 Vuetify 설정 - Setup Firestore
Firestore 설정 - Setup Vuex Store with Modules
모듈을 사용하여 Vuex Store 설정 - Create Components for Writing and Viewing Posts
게시물 작성 및 조회를 위한 구성요소 생성 - Integrate Components with Vuex and Firestore
Vuex 및 Firestore와 구성 요소 통합
1. Setup Vue and Vuetify
First, ensure you have a Vue project set up with Vuetify.
먼저 Vuetify로 Vue 프로젝트가 설정되어 있는지 확인하세요.
vue create my-blog cd my-blog vue add vuetify
2. Setup Firestore
Make sure you have Firebase set up and Firestore enabled in your Firebase project. Install Firebase in your Vue project:
Firebase 프로젝트에서 Firebase가 설정되어 있고 Firestore가 활성화되어 있는지 확인하세요. Vue 프로젝트에 Firebase를 설치합니다.
npm install firebase
Create a file firebase.js for Firebase configuration.
Firebase 구성을 위해 'firebase.js' 파일을 만듭니다.
firebase.js
import firebase from 'firebase/app';
import 'firebase/firestore';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
firebase.initializeApp(firebaseConfig);
const db = firebase.firestore();
export { db };
3. Setup Vuex Store with Modules
Create Vuex modules for posts.
게시물에 대한 Vuex 모듈을 만듭니다.
store/modules/posts.js
import { db } from '../../firebase';
const state = {
posts: [],
loading: false,
error: null
};
const mutations = {
setLoading(state, loading) {
state.loading = loading;
},
setPosts(state, posts) {
state.posts = posts;
},
setError(state, error) {
state.error = error;
}
};
const actions = {
async fetchPosts({ commit }) {
commit('setLoading', true);
try {
const postsSnapshot = await db.collection('posts').get();
const posts = [];
postsSnapshot.forEach(doc => {
posts.push({ id: doc.id, ...doc.data() });
});
commit('setPosts', posts);
} catch (error) {
commit('setError', error);
} finally {
commit('setLoading', false);
}
},
async addPost({ commit, dispatch }, post) {
commit('setLoading', true);
try {
await db.collection('posts').add(post);
dispatch('fetchPosts');
} catch (error) {
commit('setError', error);
} finally {
commit('setLoading', false);
}
}
};
export default {
namespaced: true,
state,
mutations,
actions
};
store/index.js
import Vue from 'vue';
import Vuex from 'vuex';
import posts from './modules/posts';
Vue.use(Vuex);
export default new Vuex.Store({
modules: {
posts
}
});
4. Create Components for Writing and Viewing Posts
components/PostForm.vue
<template>
<v-form @submit.prevent="submitPost">
<v-text-field v-model="title" label="Title" required></v-text-field>
<v-textarea v-model="content" label="Content" required></v-textarea>
<v-btn type="submit" color="primary">Submit</v-btn>
</v-form>
</template>
<script>
export default {
data() {
return {
title: '',
content: ''
};
},
methods: {
submitPost() {
const post = {
title: this.title,
content: this.content,
createdAt: new Date()
};
this.$store.dispatch('posts/addPost', post);
this.title = '';
this.content = '';
}
}
};
</script>
components/PostList.vue
<template>
<v-container>
<v-alert v-if="error" type="error" dismissible>{{ error.message }}</v-alert>
<v-progress-circular v-if="loading" indeterminate></v-progress-circular>
<v-list v-if="!loading">
<v-list-item v-for="post in posts" :key="post.id">
<v-list-item-content>
<v-list-item-title>{{ post.title }}</v-list-item-title>
<v-list-item-subtitle>{{ post.content }}</v-list-item-subtitle>
</v-list-item-content>
</v-list-item>
</v-list>
</v-container>
</template>
<script>
export default {
computed: {
posts() {
return this.$store.state.posts.posts;
},
loading() {
return this.$store.state.posts.loading;
},
error() {
return this.$store.state.posts.error;
}
},
created() {
this.$store.dispatch('posts/fetchPosts');
}
};
</script>
5. Integrate Components with Vuex and Firestore
Include these components in your main App.vue file or a specific route if using Vue Router.
Vue Router를 사용하는 경우 기본 App.vue 파일 또는 특정 경로에 이러한 구성 요소를 포함합니다.
App.vue
<template>
<v-app>
<v-app-bar app>
<v-toolbar-title>My Blog</v-toolbar-title>
</v-app-bar>
<v-main>
<v-container>
<post-form></post-form>
<post-list></post-list>
</v-container>
</v-main>
</v-app>
</template>
<script>
import PostForm from './components/PostForm.vue';
import PostList from './components/PostList.vue';
export default {
components: {
PostForm,
PostList
}
};
</script>
Explanation 설명
- Firestore Setup: Initialize Firestore with Firebase configuration.
Firestore 설정: Firebase 구성으로 Firestore를 초기화합니다. - Vuex Modules: Create Vuex modules for posts, including actions to fetch and add posts.
Vuex 모듈: 게시물을 가져오고 추가하는 작업을 포함하여 게시물에 대한 Vuex 모듈을 만듭니다. - Components:
- PostForm.vue: A form to submit new blog posts.
PostForm.vue: 새 블로그 게시물을 제출하기 위한 양식입니다. - PostList.vue: A component to display the list of blog posts.
PostList.vue: 블로그 게시물 목록을 표시하는 구성 요소입니다.
- PostForm.vue: A form to submit new blog posts.
- App.vue: Integrate the components into your main application.
App.vue: 구성 요소를 기본 애플리케이션에 통합합니다.
Additional Considerations 추가 고려사항
- Authentication: You may want to add authentication to ensure only authorized users can add posts.
인증: 승인된 사용자만 게시물을 추가할 수 있도록 인증을 추가할 수 있습니다. - Error Handling: Enhance error handling for a better user experience.
오류 처리: 더 나은 사용자 경험을 위해 오류 처리를 강화합니다. - Styling: Customize the components with Vuetify’s extensive styling options.
스타일링: Vuetify의 광범위한 스타일링 옵션을 사용하여 구성요소를 사용자 정의하세요.
This setup provides a basic structure to write and view blog posts using Vue, Vuex, Vuetify, and Firestore. You can expand this by adding features like editing and deleting posts, user authentication, and more.
이 설정은 Vue, Vuex, Vuetify 및 Firestore를 사용하여 블로그 게시물을 작성하고 보기 위한 기본 구조를 제공합니다. 게시물 편집 및 삭제, 사용자 인증 등과 같은 기능을 추가하여 이를 확장할 수 있습니다.
ChatGPT가 시키는 대로 했습니다.
지금은 프로젝트를 진행하는 상태이므로 ChatGPT가 제시하는 예제를 진행중인 프로젝트에 어울리도록 일부 수정하여 적용됩니다. 글쓰기 기능 구현을 위한 기능을 현재 진행 중인 프로젝트에 추가하도록 하겠습니다.
1. store/modules/posts.js
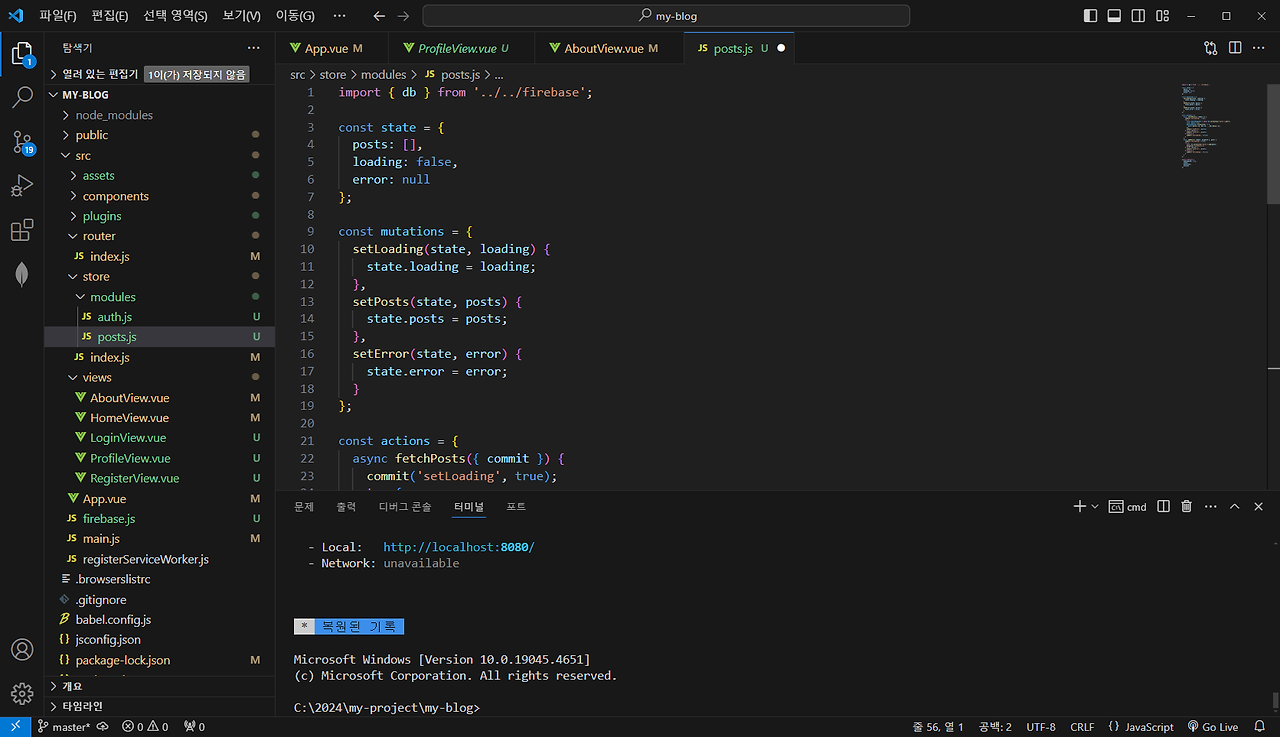
2. store/index.js
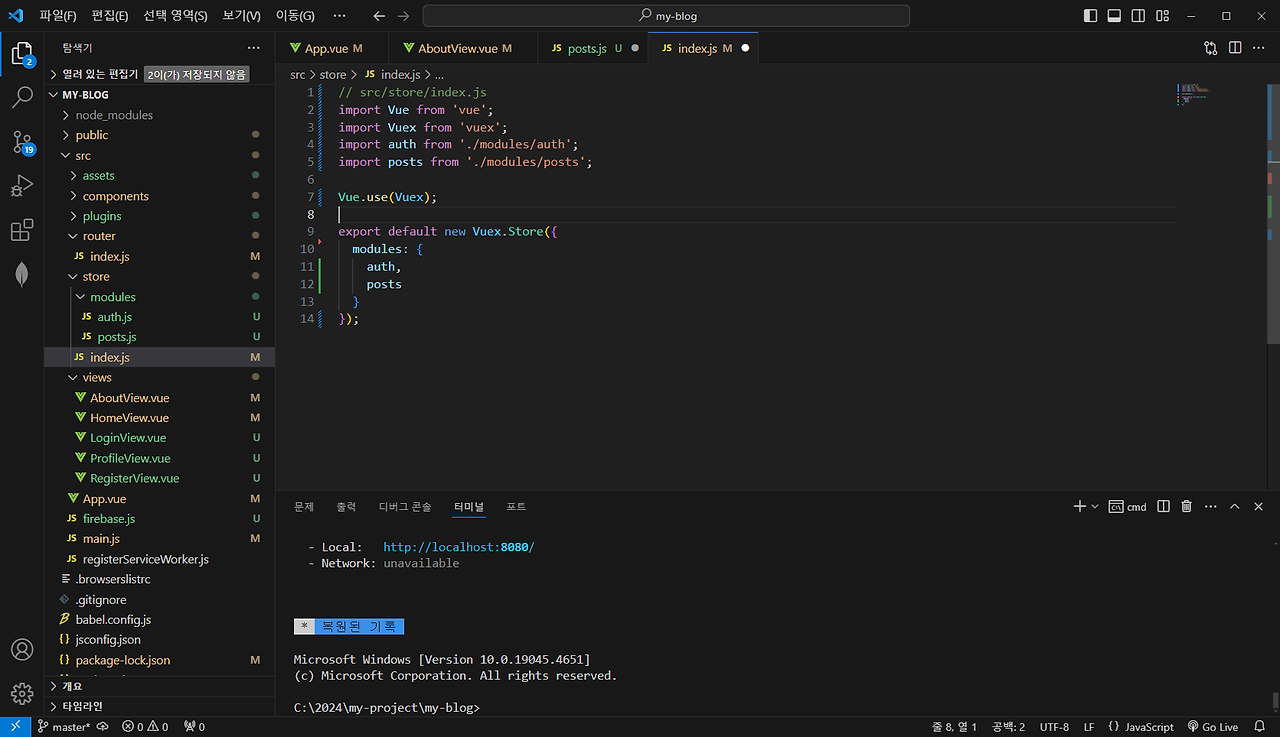
3. components/PostForm.vue
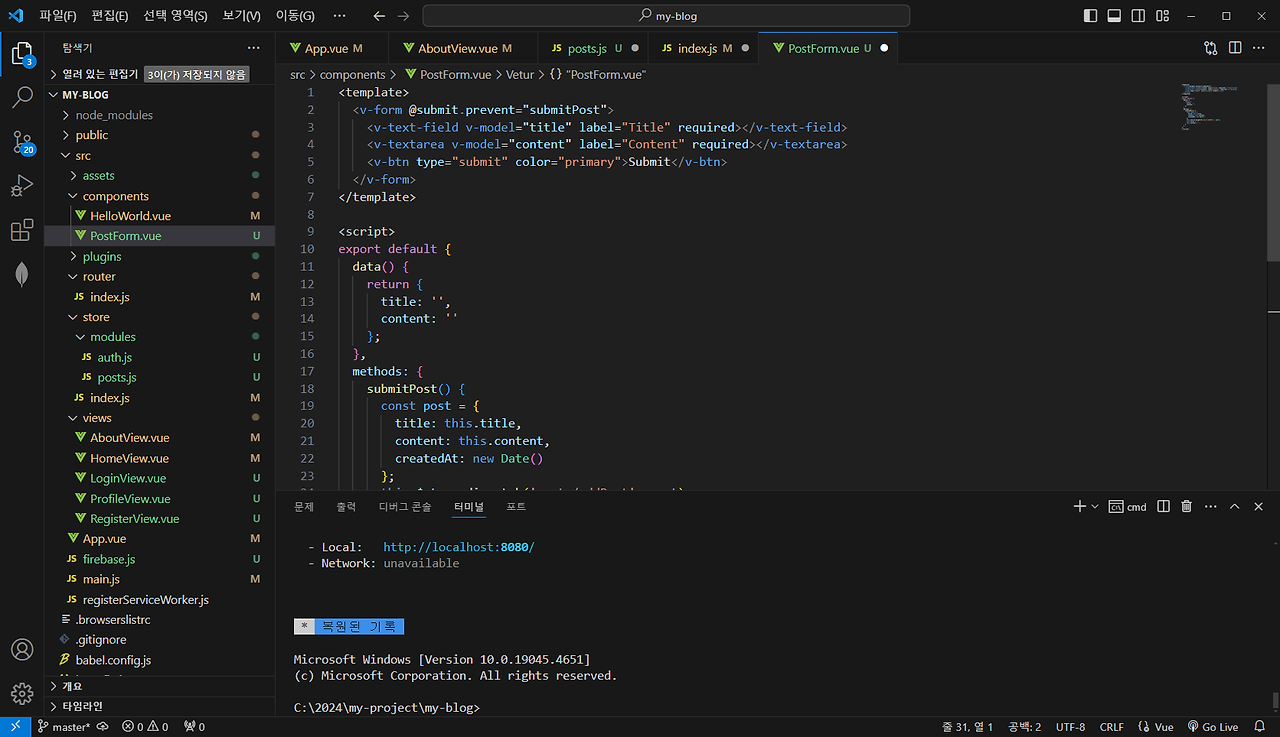
4. components/PostList.vue
이것은 HomeView.vue에 넣도록 합니다.
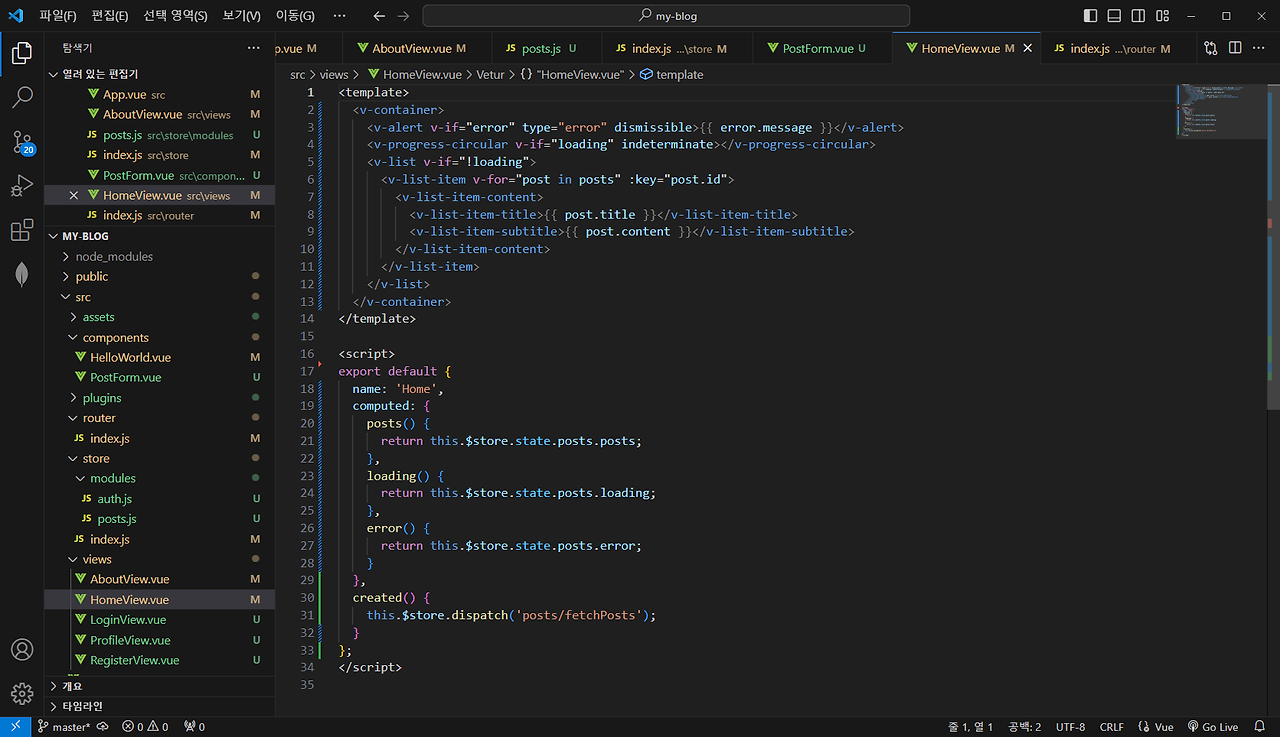
5. 실행
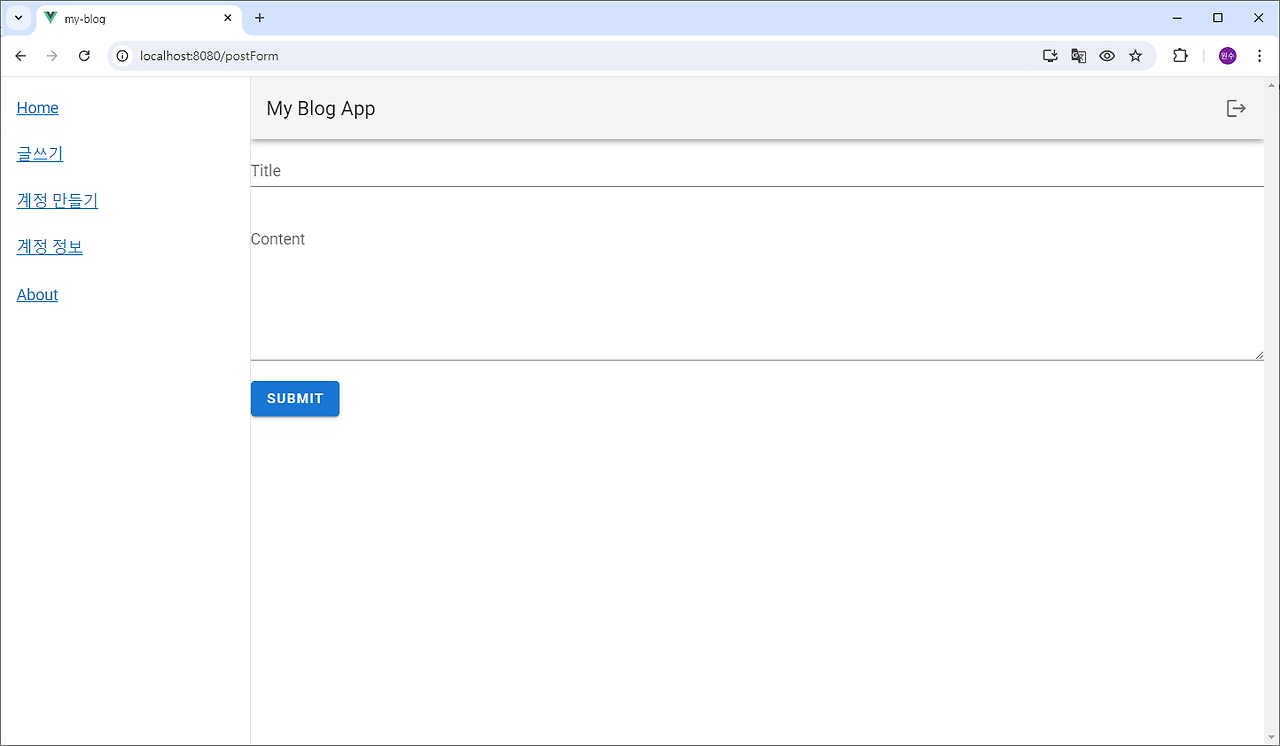
'PWA' 카테고리의 다른 글
ChatGPT와 함께 PWA Blog 개발 - 글 상세보기 HTML (0) | 2024.08.16 |
---|---|
ChatGPT와 함께 PWA Blog 개발 - 글 상세보기 (0) | 2024.08.15 |
ChatGPT와 함께 PWA Blog 개발 - 프로젝트 (1) | 2024.08.12 |
ChatGPT와 함께 PWA Blog 개발 - 설계 (0) | 2024.08.11 |
사용자 인증 - 소스코드 (0) | 2024.08.10 |