로그인 전에 접근할 수 있는 페이지와 로그인 후에 접근할수 있는 페이지가 다릅니다. 로그인한 후에만 특정 페이지에 대한 액세스를 허용합니다.
1. 로그인 전

2. 로그인 후
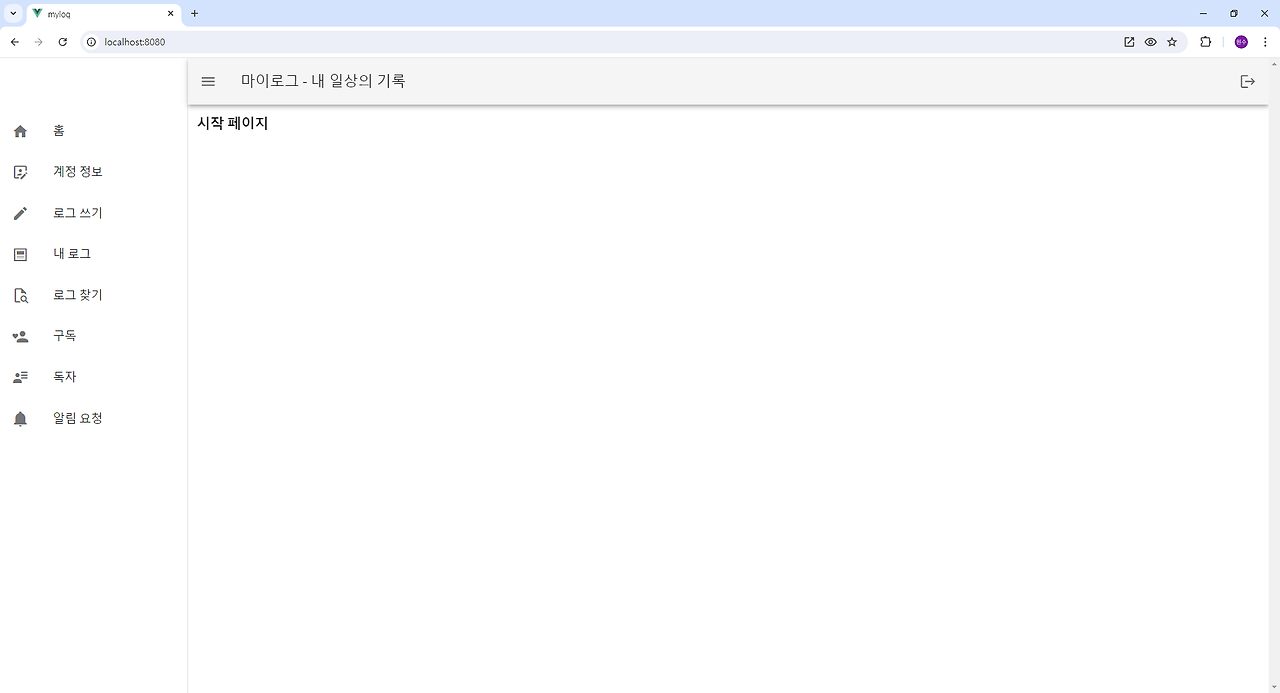
3. router
// src/router/index.js
import Vue from 'vue'
import VueRouter from 'vue-router'
import store from '../store';
import HomeView from '../views/HomeView.vue'
import LoginView from '../views/LoginView.vue'
import RegisterView from '../views/RegisterView.vue'
import ProfileView from '../views/ProfileView.vue'
import WriteMyLogView from '../views/WriteMyLogView.vue'
import MyMyLogsView from '../views/MyMyLogsView.vue'
import UserMyLogsView from '../views/UserMyLogsView.vue'
import SearchMyLogsView from '../views/SearchMyLogsView.vue'
import SubscribingView from '../views/SubscribingView.vue'
import SubscriberView from '../views/SubscriberView.vue'
import NotificationView from '../views/NotificationView.vue'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'HomeView',
component: HomeView
},
{
path: '/login',
name: 'LoginView',
component: LoginView
},
{
path: '/register',
name: 'RegisterView',
component: RegisterView
},
{
path: '/profile',
name: 'ProfileView',
component: ProfileView,
meta: { requiresAuth: true }
},
{
path: '/write',
name: 'WriteMyLogView',
component: WriteMyLogView,
meta: { requiresAuth: true }
},
{
path: '/mylogs',
name: 'MyMyLogsView',
component: MyMyLogsView,
meta: { requiresAuth: true }
},
{
path: '/userlogs:userid',
name: 'UserMyLogsView',
component: UserMyLogsView
},
{
path: '/search',
name: 'SearchMyLogsView',
component: SearchMyLogsView
},
{
path: '/subscribing',
name: 'SubscribingView',
component: SubscribingView,
meta: { requiresAuth: true }
},
{
path: '/subscriber',
name: 'SubscriberView',
component: SubscriberView,
meta: { requiresAuth: true }
},
{
path: '/notification',
name: 'NotificationView',
component: NotificationView,
meta: { requiresAuth: true }
},
{
path: '/about',
name: 'about',
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '../views/AboutView.vue')
}
]
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
})
router.beforeEach((to, from, next) => {
const requiresAuth = to.matched.some(record => record.meta.requiresAuth);
const user = store.state.auth.user;
if (requiresAuth && !user) {
next('/login');
} else {
next();
}
});
export default router
4. App.vue
<!-- src/App.vue -->
<template>
<v-app>
<!-- App Bar -->
<v-app-bar app>
<v-app-bar-nav-icon @click="drawer = !drawer"></v-app-bar-nav-icon>
<v-toolbar-title>마이로그 - 내 일상의 기록</v-toolbar-title>
<v-spacer></v-spacer>
<v-btn icon @click="doLogout" v-if="user">
<v-icon>mdi-logout</v-icon>
</v-btn>
</v-app-bar>
<!-- Navigation Drawer -->
<v-navigation-drawer app v-model="drawer" :clipped="$vuetify.breakpoint.lgAndUp">
<v-list>
<v-list-item-group>
<!-- Loop through routes array to create navigation items -->
<v-list-item v-for="(route, index) in GetMenuItems" :key="index" @click="$router.push(route.path)">
<v-list-item-icon>
<v-icon>{{ route.icon }}</v-icon>
</v-list-item-icon>
<v-list-item-content>
<v-list-item-title>{{ route.title }}</v-list-item-title>
</v-list-item-content>
</v-list-item>
</v-list-item-group>
</v-list>
</v-navigation-drawer>
<!-- Main Content with Router View -->
<v-main>
<v-container fluid>
<!-- Router View to display route components -->
<router-view></router-view>
</v-container>
</v-main>
</v-app>
</template>
<script>
import { mapActions, mapGetters } from 'vuex';
export default {
data() {
return {
drawer: true, // State to toggle the navigation drawer
};
},
computed: {
...mapGetters('auth', ['user']),
// 로그인 여부에 따라 다르게 탐색서랍과 툴바 메뉴명 항목 배열 반환
GetMenuItems() {
if(this.user) {
return [
{ title: '홈', path: '/', icon: 'mdi-home'},
{ title: '계정 정보', path: '/profile', icon: 'mdi-account-box-edit-outline'},
{ title: '로그 쓰기', path: '/write', icon: 'mdi-pencil'},
{ title: '내 로그', path: '/mylogs', icon: 'mdi-post-outline'},
{ title: '로그 찾기', path: '/search', icon: 'mdi-file-search-outline'},
{ title: '구독', path: '/subscribing', icon: 'mdi-account-heart'},
{ title: '독자', path: '/subscriber', icon: 'mdi-account-details'},
{ title: '알림 요청', path: '/notification', icon: 'mdi-bell'}
]
} else {
return [
{ title: '홈', path: '/', icon: 'mdi-home'},
{ title: '로그인', path: '/login', icon: 'mdi-login'},
{ title: '계정 만들기', path: '/register', icon: 'mdi-account'},
{ title: '로그 찾기', path: '/search', icon: 'mdi-file-search-outline'}
]
}
}
},
methods: {
...mapActions('auth', ['logout']),
doLogout() {
this.logout()
}
}
};
</script>
'Vue로 PWA 개발' 카테고리의 다른 글
16. mylog 쓰기 (2) | 2024.10.18 |
---|---|
15. mylog 리스트 (2) | 2024.10.18 |
13. mylog 페이지 - 인증 후 보기 (5) | 2024.10.12 |
12. mylog 로그인 - 완성 (0) | 2024.10.11 |
11. mylog 로그인 - google 로그인 (2) | 2024.10.11 |